实例介绍
【实例简介】
【实例截图】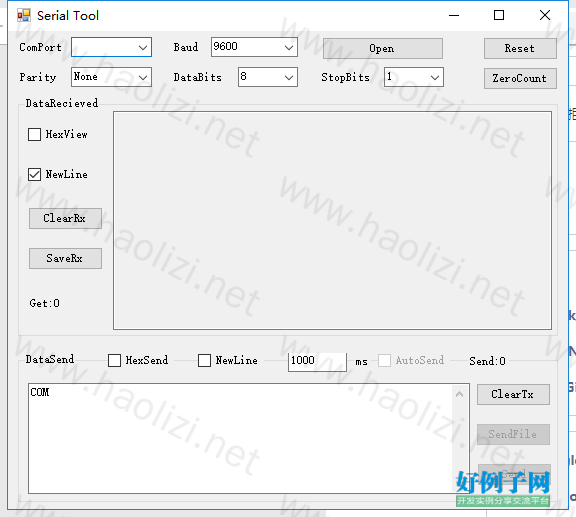
【实例截图】
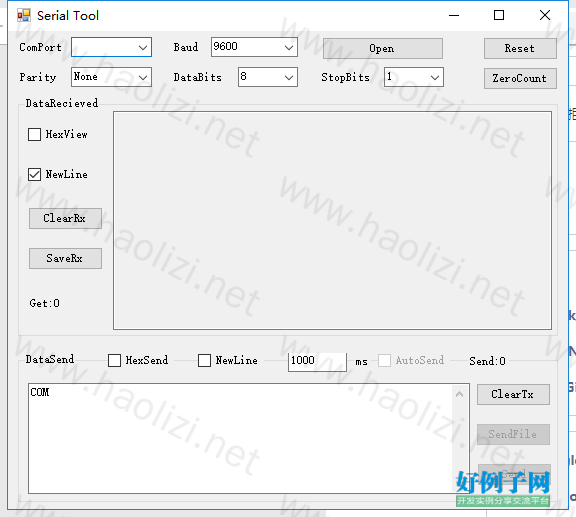
【核心代码】
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Windows.Forms; using System.IO.Ports; using System.IO; using System.Text.RegularExpressions; namespace SerialPortDemo { public partial class Form1 : Form { private SerialPort spCom = new SerialPort(); private StringBuilder strBuilderRx = new StringBuilder(); private Timer timerAutoSend = new Timer(); private long nCountRx = 0; private long nCountTx = 0; private string strFn; public Form1() { InitializeComponent(); } private void SerialPortForm_Load(object sender, EventArgs e) { string[] straPorts = SerialPort.GetPortNames(); Array.Sort(straPorts); comboPortName.Items.AddRange(straPorts); comboPortName.SelectedIndex = comboPortName.Items.Count > 0 ? 0 : -1; comboBaudRate.SelectedIndex = comboBaudRate.Items.IndexOf("9600"); comboParity.SelectedIndex = comboParity.Items.IndexOf("None"); comboDataBits.SelectedIndex = comboDataBits.Items.IndexOf("8"); comboStopBits.SelectedIndex = comboStopBits.Items.IndexOf("1"); spCom.NewLine = "\r\n"; spCom.RtsEnable = true; buttonSend.Enabled = false; checkBoxAutoSend.Enabled = false; checkBoxNewLineRx.CheckState = CheckState.Checked; buttonSendFile.Enabled = false; comboPortName.Enabled = true; comboBaudRate.Enabled = true; comboParity.Enabled = true; comboDataBits.Enabled = true; comboStopBits.Enabled = true; textBoxTx.Text = "COM"; textBoxTimerMs.Text = "1000"; spCom.DataReceived = new SerialDataReceivedEventHandler(Com_DataReceived); timerAutoSend.Tick = new EventHandler(AutoSendTimer_Tick); } void AutoSendTimer_Tick(object sender, EventArgs e) { buttonSend_Click(sender, e); } void Com_DataReceived(object sender, SerialDataReceivedEventArgs e) { int nReadSum = spCom.BytesToRead; nCountRx = nReadSum; byte[] byteaBuf = new byte[nReadSum]; spCom.Read(byteaBuf, 0, nReadSum); strBuilderRx.Clear(); //因为要访问ui资源,所以需要使用invoke方式同步ui this.Invoke((EventHandler)(delegate { if (checkBoxHexView.Checked) { foreach (byte byteBit in byteaBuf) { strBuilderRx.Append(byteBit.ToString("X2") " "); } } else { strBuilderRx.Append(Encoding.ASCII.GetString(byteaBuf)); } this.textBoxRx.AppendText(strBuilderRx.ToString()); labelSumRx.Text = "Get:" nCountRx.ToString(); })); } private void buttonOpen_Click(object sender, EventArgs e) { if (spCom.IsOpen) { spCom.Close(); } else { spCom.PortName = comboPortName.Text; spCom.BaudRate = int.Parse(comboBaudRate.Text); switch (comboParity.Text) { case "None": spCom.Parity = Parity.None; break; case "Odd": spCom.Parity = Parity.Odd; break; case "Even": spCom.Parity = Parity.Even; break; case "Mark": spCom.Parity = Parity.Mark; break; case "Space": spCom.Parity = Parity.Space; break; default: spCom.Parity = Parity.None; break; } spCom.DataBits = int.Parse(comboDataBits.Text); switch (comboStopBits.Text) { case "1": spCom.StopBits = StopBits.One; break; case "1.5": spCom.StopBits = StopBits.OnePointFive; break; case "2": spCom.StopBits = StopBits.Two; break; default: spCom.StopBits = StopBits.One; break; } try { spCom.Open(); } catch (System.Exception ex) { spCom = new SerialPort(); MessageBox.Show(ex.Message); } } buttonOpen.Text = spCom.IsOpen ? "Close" : "Open"; buttonSend.Enabled = spCom.IsOpen; checkBoxAutoSend.Enabled = spCom.IsOpen; buttonSendFile.Enabled = spCom.IsOpen; comboPortName.Enabled = !spCom.IsOpen; comboBaudRate.Enabled = !spCom.IsOpen; comboParity.Enabled = !spCom.IsOpen; comboDataBits.Enabled = !spCom.IsOpen; comboStopBits.Enabled = !spCom.IsOpen; } private void checkBoxNewLineRx_CheckedChanged(object sender, EventArgs e) { textBoxRx.WordWrap = checkBoxNewLineRx.Checked; } private void buttonSend_Click(object sender, EventArgs e) { int nSendSum = 0; if (checkBoxHexSend.Checked) { MatchCollection mc = Regex.Matches(textBoxTx.Text, @"(?i)[\da-f]{2}"); List<byte> buf = new List<byte>(); foreach (Match m in mc) { buf.Add(byte.Parse(m.Value)); } spCom.Write(buf.ToArray(), 0, buf.Count); nSendSum = buf.Count; } else { if (checkBoxNewLineTx.Checked) { spCom.WriteLine(textBoxTx.Text); nSendSum = textBoxTx.Text.Length 2; } else { spCom.Write(textBoxTx.Text); nSendSum = textBoxTx.Text.Length; } } nCountTx = nSendSum; labelSumTx.Text = "Send:" nCountTx.ToString(); } private void buttonReset_Click(object sender, EventArgs e) { nCountTx = nCountRx = 0; labelSumRx.Text = "Get:0"; labelSumTx.Text = "Send:0"; checkBoxHexView.CheckState = CheckState.Unchecked; checkBoxNewLineRx.CheckState = CheckState.Checked; checkBoxHexSend.CheckState = CheckState.Unchecked; checkBoxNewLineTx.CheckState = CheckState.Unchecked; checkBoxAutoSend.CheckState = CheckState.Unchecked; textBoxRx.Clear(); textBoxTx.Clear(); } private void buttonZeroCount_Click(object sender, EventArgs e) { nCountTx = nCountRx = 0; labelSumTx.Text = "Send:" nCountTx.ToString(); labelSumRx.Text = "Get:" nCountRx.ToString(); } private void buttonClearRx_Click(object sender, EventArgs e) { textBoxRx.Clear(); } private void AutoSend(object sender, EventArgs e) { if (checkBoxAutoSend.Checked == true) { timerAutoSend.Interval = Convert.ToInt32(textBoxTimerMs.Text, 10); timerAutoSend.Start(); } else { timerAutoSend.Stop(); } } private void Timer_ms_KeyPress(object sender, KeyPressEventArgs e) { if ((e.KeyChar < 48 || e.KeyChar > 57) && e.KeyChar != 8) { e.Handled = true; } } private void buttonSaveRx_Click(object sender, EventArgs e) { if (dlgSaveFile.ShowDialog() == DialogResult.OK) { strFn = dlgSaveFile.FileName; try { File.WriteAllText(strFn, textBoxRx.Text); } catch (System.Exception ex) { MessageBox.Show(ex.Message, "SerialPort Tool error", MessageBoxButtons.OK, MessageBoxIcon.Exclamation); } } } private void buttonClearTx_Click(object sender, EventArgs e) { textBoxTx.Clear(); } private void buttonSendFile_Click(object sender, EventArgs e) { if (dlgOpenFile.ShowDialog() == DialogResult.OK) { strFn = dlgOpenFile.FileName; try { spCom.Write(File.ReadAllText(strFn)); nCountTx = File.ReadAllText(strFn).Length; labelSumTx.Text = "Send:" nCountTx.ToString(); } catch (System.Exception ex) { MessageBox.Show(ex.Message, "SerialPort Error", MessageBoxButtons.OK, MessageBoxIcon.Exclamation); } } } } }
好例子网口号:伸出你的我的手 — 分享!
网友评论
小贴士
感谢您为本站写下的评论,您的评论对其它用户来说具有重要的参考价值,所以请认真填写。
- 类似“顶”、“沙发”之类没有营养的文字,对勤劳贡献的楼主来说是令人沮丧的反馈信息。
- 相信您也不想看到一排文字/表情墙,所以请不要反馈意义不大的重复字符,也请尽量不要纯表情的回复。
- 提问之前请再仔细看一遍楼主的说明,或许是您遗漏了。
- 请勿到处挖坑绊人、招贴广告。既占空间让人厌烦,又没人会搭理,于人于己都无利。
关于好例子网
本站旨在为广大IT学习爱好者提供一个非营利性互相学习交流分享平台。本站所有资源都可以被免费获取学习研究。本站资源来自网友分享,对搜索内容的合法性不具有预见性、识别性、控制性,仅供学习研究,请务必在下载后24小时内给予删除,不得用于其他任何用途,否则后果自负。基于互联网的特殊性,平台无法对用户传输的作品、信息、内容的权属或合法性、安全性、合规性、真实性、科学性、完整权、有效性等进行实质审查;无论平台是否已进行审查,用户均应自行承担因其传输的作品、信息、内容而可能或已经产生的侵权或权属纠纷等法律责任。本站所有资源不代表本站的观点或立场,基于网友分享,根据中国法律《信息网络传播权保护条例》第二十二与二十三条之规定,若资源存在侵权或相关问题请联系本站客服人员,点此联系我们。关于更多版权及免责申明参见 版权及免责申明
支持(0) 盖楼(回复)