实例介绍
c#Winform自定义控件-基类控件,按钮,有图标的按钮,选择按钮组,复选框,单选框,进度条,分割线,树,横向列表,列表,分页控件,导航菜单,键盘,文本框,表格,日期控件,Tab页,下拉框,步骤控件,有标题的面板,圆形进度条,面包屑导航,开关等等。
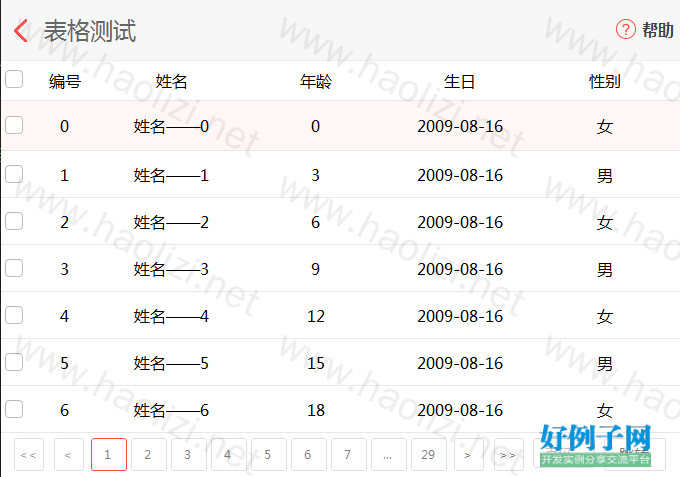
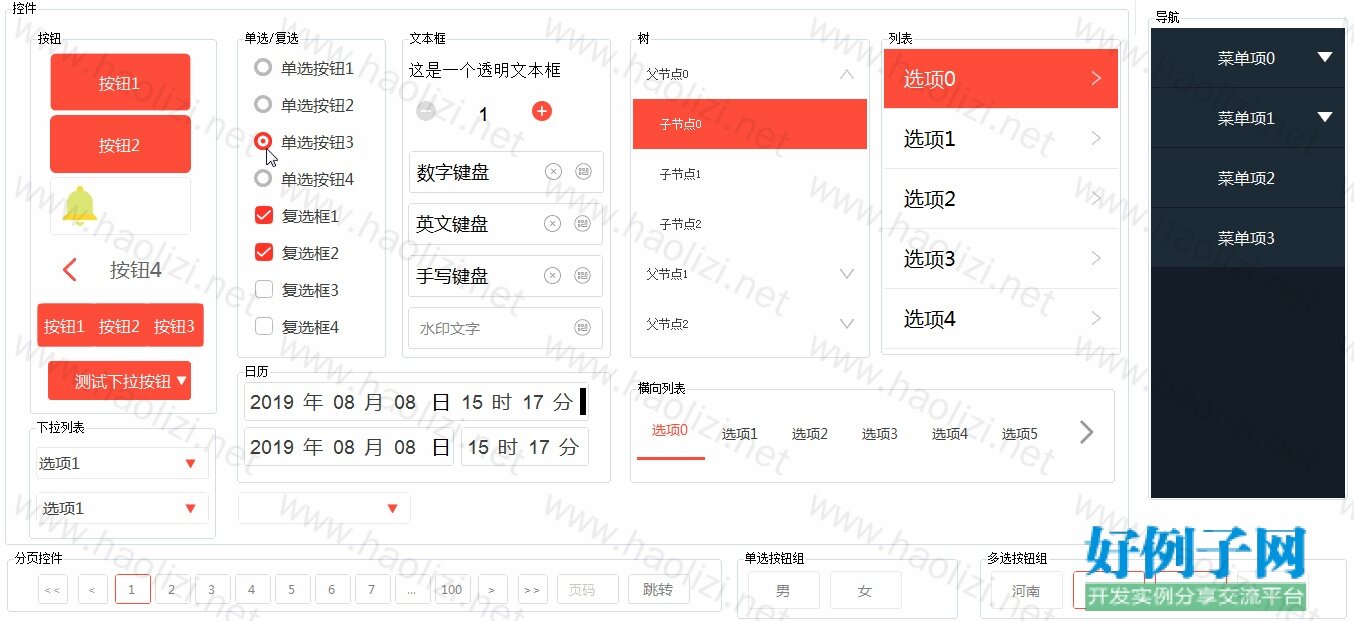
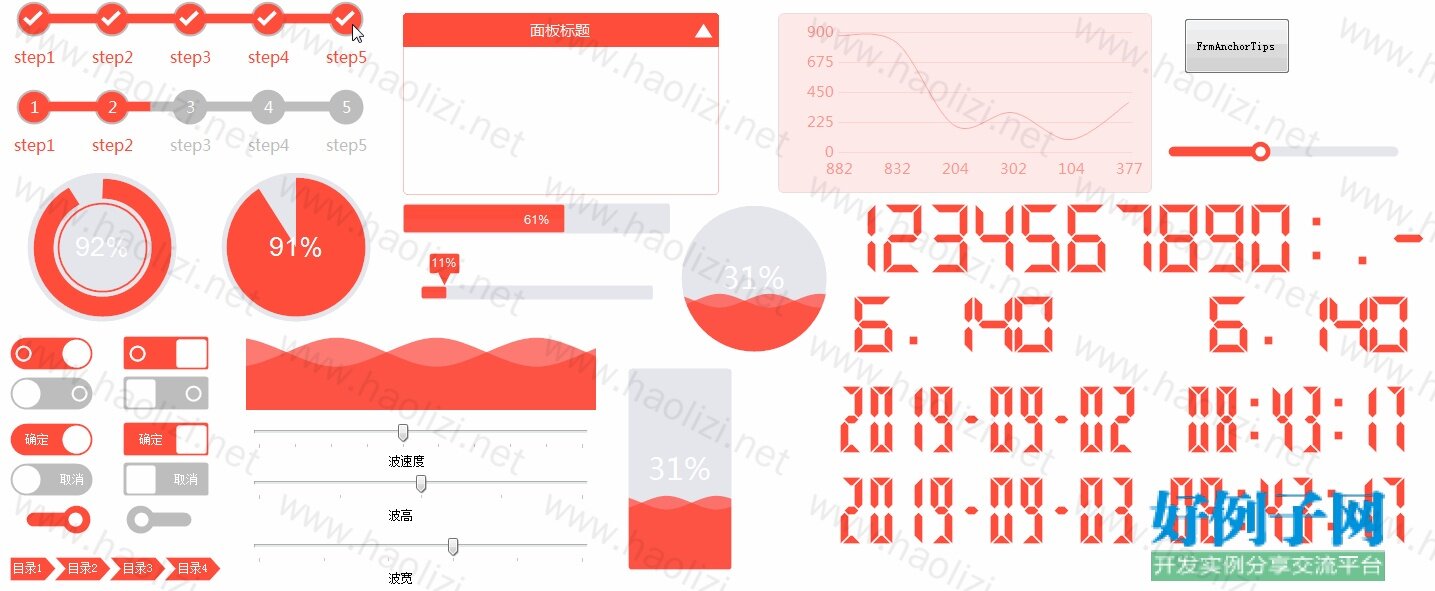
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Drawing;
using System.Data;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace HZH_Controls.Controls
{
/// <summary>
/// Class UCBtnsGroup.
/// Implements the <see cref="System.Windows.Forms.UserControl" />
/// </summary>
/// <seealso cref="System.Windows.Forms.UserControl" />
public partial class UCBtnsGroup : UserControl
{
/// <summary>
/// 选中改变事件
/// </summary>
[Description("选中改变事件"), Category("自定义")]
public event EventHandler SelectedItemChanged;
/// <summary>
/// The m data source
/// </summary>
private Dictionary<string, string> m_dataSource = new Dictionary<string, string>();
/// <summary>
/// 数据源
/// </summary>
/// <value>The data source.</value>
[Description("数据源"), Category("自定义")]
public Dictionary<string, string> DataSource
{
get { return m_dataSource; }
set
{
m_dataSource = value;
Reload();
}
}
/// <summary>
/// The m select item
/// </summary>
private List<string> m_selectItem = new List<string>();
/// <summary>
/// 选中项
/// </summary>
/// <value>The select item.</value>
[Description("选中项"), Category("自定义")]
public List<string> SelectItem
{
get { return m_selectItem; }
set
{
m_selectItem = value;
if (m_selectItem == null)
m_selectItem = new List<string>();
SetSelected();
}
}
/// <summary>
/// The m is multiple
/// </summary>
private bool m_isMultiple = false;
/// <summary>
/// 是否多选
/// </summary>
/// <value><c>true</c> if this instance is multiple; otherwise, <c>false</c>.</value>
[Description("是否多选"), Category("自定义")]
public bool IsMultiple
{
get { return m_isMultiple; }
set { m_isMultiple = value; }
}
/// <summary>
/// Initializes a new instance of the <see cref="UCBtnsGroup" /> class.
/// </summary>
public UCBtnsGroup()
{
InitializeComponent();
}
/// <summary>
/// Reloads this instance.
/// </summary>
private void Reload()
{
try
{
ControlHelper.FreezeControl(flowLayoutPanel1, true);
this.flowLayoutPanel1.Controls.Clear();
if (DataSource != null)
{
foreach (var item in DataSource)
{
UCBtnExt btn = new UCBtnExt();
btn.BackColor = System.Drawing.Color.Transparent;
btn.BtnBackColor = System.Drawing.Color.White;
btn.BtnFont = new System.Drawing.Font("微软雅黑", 10F);
btn.BtnForeColor = System.Drawing.Color.Gray;
btn.BtnText = item.Value;
btn.ConerRadius = 5;
btn.Cursor = System.Windows.Forms.Cursors.Hand;
btn.FillColor = System.Drawing.Color.White;
btn.Font = new System.Drawing.Font("微软雅黑", 15F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Pixel);
btn.IsRadius = true;
btn.IsShowRect = true;
btn.IsShowTips = false;
btn.Location = new System.Drawing.Point(5, 5);
btn.Margin = new System.Windows.Forms.Padding(5);
btn.Name = item.Key;
btn.RectColor = System.Drawing.Color.FromArgb(224, 224, 224);
btn.RectWidth = 1;
btn.Size = new System.Drawing.Size(72, 38);
btn.TabStop = false;
btn.BtnClick = btn_BtnClick;
this.flowLayoutPanel1.Controls.Add(btn);
}
}
}
finally
{
ControlHelper.FreezeControl(flowLayoutPanel1, false);
}
SetSelected();
}
/// <summary>
/// Handles the BtnClick event of the btn control.
/// </summary>
/// <param name="sender">The source of the event.</param>
/// <param name="e">The <see cref="EventArgs" /> instance containing the event data.</param>
void btn_BtnClick(object sender, EventArgs e)
{
var btn = sender as UCBtnExt;
if (m_selectItem.Contains(btn.Name))
{
btn.RectColor = System.Drawing.Color.FromArgb(224, 224, 224);
m_selectItem.Remove(btn.Name);
}
else
{
if (!m_isMultiple)
{
foreach (var item in m_selectItem)
{
var lst = this.flowLayoutPanel1.Controls.Find(item, false);
if (lst.Length == 1)
{
var _btn = lst[0] as UCBtnExt;
_btn.RectColor = System.Drawing.Color.FromArgb(224, 224, 224);
}
}
m_selectItem.Clear();
}
btn.RectColor = System.Drawing.Color.FromArgb(255, 77, 59);
m_selectItem.Add(btn.Name);
}
if (SelectedItemChanged != null)
SelectedItemChanged(this, e);
}
/// <summary>
/// Sets the selected.
/// </summary>
private void SetSelected()
{
if (m_selectItem != null && m_selectItem.Count > 0 && DataSource != null && DataSource.Count > 0)
{
try
{
ControlHelper.FreezeControl(flowLayoutPanel1, true);
if (m_isMultiple)
{
foreach (var item in m_selectItem)
{
var lst = this.flowLayoutPanel1.Controls.Find(item, false);
if (lst.Length == 1)
{
var btn = lst[0] as UCBtnExt;
btn.RectColor = System.Drawing.Color.FromArgb(255, 77, 59);
}
}
}
else
{
UCBtnExt btn = null;
foreach (var item in m_selectItem)
{
var lst = this.flowLayoutPanel1.Controls.Find(item, false);
if (lst.Length == 1)
{
btn = lst[0] as UCBtnExt;
break;
}
}
if (btn != null)
{
m_selectItem = new List<string>() { btn.Name };
btn.RectColor = System.Drawing.Color.FromArgb(255, 77, 59);
}
}
}
finally
{
ControlHelper.FreezeControl(flowLayoutPanel1, false);
}
}
}
}
}
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Drawing;
using System.Data;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Drawing.Drawing2D;
namespace HZH_Controls.Controls
{
/// <summary>
/// Class UCStep.
/// Implements the <see cref="System.Windows.Forms.UserControl" />
/// </summary>
/// <seealso cref="System.Windows.Forms.UserControl" />
[DefaultEvent("IndexChecked")]
public partial class UCStep : UserControl
{
/// <summary>
/// Occurs when [index checked].
/// </summary>
[Description("步骤更改事件"), Category("自定义")]
public event EventHandler IndexChecked;
/// <summary>
/// The m step back color
/// </summary>
private Color m_stepBackColor = Color.FromArgb(189, 189, 189);
/// <summary>
/// 步骤背景色
/// </summary>
/// <value>The color of the step back.</value>
[Description("步骤背景色"), Category("自定义")]
public Color StepBackColor
{
get { return m_stepBackColor; }
set
{
m_stepBackColor = value;
Refresh();
}
}
/// <summary>
/// The m step fore color
/// </summary>
private Color m_stepForeColor = Color.FromArgb(255, 77, 59);
/// <summary>
/// 步骤前景色
/// </summary>
/// <value>The color of the step fore.</value>
[Description("步骤前景色"), Category("自定义")]
public Color StepForeColor
{
get { return m_stepForeColor; }
set
{
m_stepForeColor = value;
Refresh();
}
}
/// <summary>
/// The m step font color
/// </summary>
private Color m_stepFontColor = Color.White;
/// <summary>
/// 步骤文字颜色
/// </summary>
/// <value>The color of the step font.</value>
[Description("步骤文字景色"), Category("自定义")]
public Color StepFontColor
{
get { return m_stepFontColor; }
set
{
m_stepFontColor = value;
Refresh();
}
}
/// <summary>
/// The m step width
/// </summary>
private int m_stepWidth = 35;
/// <summary>
/// 步骤宽度
/// </summary>
/// <value>The width of the step.</value>
[Description("步骤宽度景色"), Category("自定义")]
public int StepWidth
{
get { return m_stepWidth; }
set
{
m_stepWidth = value;
Refresh();
}
}
/// <summary>
/// The m steps
/// </summary>
private string[] m_steps = new string[] { "step1", "step2", "step3" };
/// <summary>
/// Gets or sets the steps.
/// </summary>
/// <value>The steps.</value>
[Description("步骤"), Category("自定义")]
public string[] Steps
{
get { return m_steps; }
set
{
if (m_steps == null || m_steps.Length <= 1)
return;
m_steps = value;
Refresh();
}
}
/// <summary>
/// The m step index
/// </summary>
private int m_stepIndex = 0;
/// <summary>
/// Gets or sets the index of the step.
/// </summary>
/// <value>The index of the step.</value>
[Description("步骤位置"), Category("自定义")]
public int StepIndex
{
get { return m_stepIndex; }
set
{
if (value > Steps.Length)
return;
m_stepIndex = value;
Refresh();
if (IndexChecked != null)
{
IndexChecked(this, null);
}
}
}
/// <summary>
/// The m line width
/// </summary>
private int m_lineWidth = 2;
/// <summary>
/// Gets or sets the width of the line.
/// </summary>
/// <value>The width of the line.</value>
[Description("连接线宽度,最小2"), Category("自定义")]
public int LineWidth
{
get { return m_lineWidth; }
set
{
if (value < 2)
return;
m_lineWidth = value;
Refresh();
}
}
/// <summary>
/// The m img completed
/// </summary>
private Image m_imgCompleted = null;
/// <summary>
/// Gets or sets the img completed.
/// </summary>
/// <value>The img completed.</value>
[Description("已完成步骤图片,当不为空时,已完成步骤将不再显示数字,建议24*24大小"), Category("自定义")]
public Image ImgCompleted
{
get { return m_imgCompleted; }
set
{
m_imgCompleted = value;
Refresh();
}
}
/// <summary>
/// The m LST cache rect
/// </summary>
List<Rectangle> m_lstCacheRect = new List<Rectangle>();
/// <summary>
/// Initializes a new instance of the <see cref="UCStep" /> class.
/// </summary>
public UCStep()
{
InitializeComponent();
this.SetStyle(ControlStyles.AllPaintingInWmPaint, true);
this.SetStyle(ControlStyles.DoubleBuffer, true);
this.SetStyle(ControlStyles.ResizeRedraw, true);
this.SetStyle(ControlStyles.Selectable, true);
this.SetStyle(ControlStyles.SupportsTransparentBackColor, true);
this.SetStyle(ControlStyles.UserPaint, true);
this.MouseDown = UCStep_MouseDown;
}
/// <summary>
/// Handles the MouseDown event of the UCStep control.
/// </summary>
/// <param name="sender">The source of the event.</param>
/// <param name="e">The <see cref="MouseEventArgs" /> instance containing the event data.</param>
void UCStep_MouseDown(object sender, MouseEventArgs e)
{
var index = m_lstCacheRect.FindIndex(p => p.Contains(e.Location));
if (index >= 0)
{
StepIndex = index 1;
}
}
/// <summary>
/// 引发 <see cref="E:System.Windows.Forms.Control.Paint" /> 事件。
/// </summary>
/// <param name="e">包含事件数据的 <see cref="T:System.Windows.Forms.PaintEventArgs" />。</param>
protected override void OnPaint(PaintEventArgs e)
{
base.OnPaint(e);
var g = e.Graphics;
g.SetGDIHigh();
if (m_steps != null && m_steps.Length > 0)
{
System.Drawing.SizeF sizeFirst = g.MeasureString(m_steps[0], this.Font);
int y = (this.Height - m_stepWidth - 10 - (int)sizeFirst.Height) / 2;
if (y < 0)
y = 0;
int intTxtY = y m_stepWidth 10;
int intLeft = 0;
if (sizeFirst.Width > m_stepWidth)
{
intLeft = (int)(sizeFirst.Width - m_stepWidth) / 2 1;
}
int intRight = 0;
System.Drawing.SizeF sizeEnd = g.MeasureString(m_steps[m_steps.Length - 1], this.Font);
if (sizeEnd.Width > m_stepWidth)
{
intRight = (int)(sizeEnd.Width - m_stepWidth) / 2 1;
}
int intSplitWidth = 20;
intSplitWidth = (this.Width - m_steps.Length - (m_steps.Length * m_stepWidth) - intRight - intLeft) / (m_steps.Length - 1);
if (intSplitWidth < 20)
intSplitWidth = 20;
m_lstCacheRect = new List<Rectangle>();
for (int i = 0; i < m_steps.Length; i )
{
#region 画圆,横线
Rectangle rectEllipse = new Rectangle(new Point(intLeft i * (m_stepWidth intSplitWidth), y), new Size(m_stepWidth, m_stepWidth));
m_lstCacheRect.Add(rectEllipse);
g.FillEllipse(new SolidBrush(m_stepBackColor), rectEllipse);
if (m_stepIndex > i)
{
g.FillEllipse(new SolidBrush(m_stepForeColor), new Rectangle(new Point(intLeft i * (m_stepWidth intSplitWidth) 2, y 2), new Size(m_stepWidth - 4, m_stepWidth - 4)));
}
if (m_stepIndex > i && m_imgCompleted != null)
{
g.DrawImage(m_imgCompleted, new Rectangle(new Point((intLeft i * (m_stepWidth intSplitWidth) (m_stepWidth - 24) / 2), y (m_stepWidth - 24) / 2), new Size(24, 24)), 0, 0, m_imgCompleted.Width, m_imgCompleted.Height, GraphicsUnit.Pixel, null);
}
else
{
System.Drawing.SizeF _numSize = g.MeasureString((i 1).ToString(), this.Font);
g.DrawString((i 1).ToString(), Font, new SolidBrush(m_stepFontColor), new Point(intLeft i * (m_stepWidth intSplitWidth) (m_stepWidth - (int)_numSize.Width) / 2 1, y (m_stepWidth - (int)_numSize.Height) / 2 1));
}
#endregion
System.Drawing.SizeF sizeTxt = g.MeasureString(m_steps[i], this.Font);
g.DrawString(m_steps[i], Font, new SolidBrush(m_stepIndex > i ? m_stepForeColor : m_stepBackColor), new Point(intLeft i * (m_stepWidth intSplitWidth) (m_stepWidth - (int)sizeTxt.Width) / 2 1, intTxtY));
}
for (int i = 0; i < m_steps.Length; i )
{
if (m_stepIndex > i)
{
if (i != m_steps.Length - 1)
{
if (m_stepIndex == i 1)
{
g.DrawLine(new Pen(m_stepForeColor, m_lineWidth), new Point(intLeft i * (m_stepWidth intSplitWidth) m_stepWidth - 3, y ((m_stepWidth) / 2)), new Point(intLeft i * (m_stepWidth intSplitWidth) m_stepWidth intSplitWidth / 2, y ((m_stepWidth) / 2)));
g.DrawLine(new Pen(m_stepBackColor, m_lineWidth), new Point(intLeft i * (m_stepWidth intSplitWidth) m_stepWidth intSplitWidth / 2, y ((m_stepWidth) / 2)), new Point(intLeft (i 1) * (m_stepWidth intSplitWidth) 10, y ((m_stepWidth) / 2)));
}
else
{
g.DrawLine(new Pen(m_stepForeColor, m_lineWidth), new Point(intLeft i * (m_stepWidth intSplitWidth) m_stepWidth - 3, y ((m_stepWidth) / 2)), new Point(intLeft (i 1) * (m_stepWidth intSplitWidth) 10, y ((m_stepWidth) / 2)));
}
}
}
else
{
if (i != m_steps.Length - 1)
{
g.DrawLine(new Pen(m_stepBackColor, m_lineWidth), new Point(intLeft i * (m_stepWidth intSplitWidth) m_stepWidth - 3, y ((m_stepWidth) / 2)), new Point(intLeft (i 1) * (m_stepWidth intSplitWidth) 10, y ((m_stepWidth) / 2)));
}
}
}
}
}
}
}
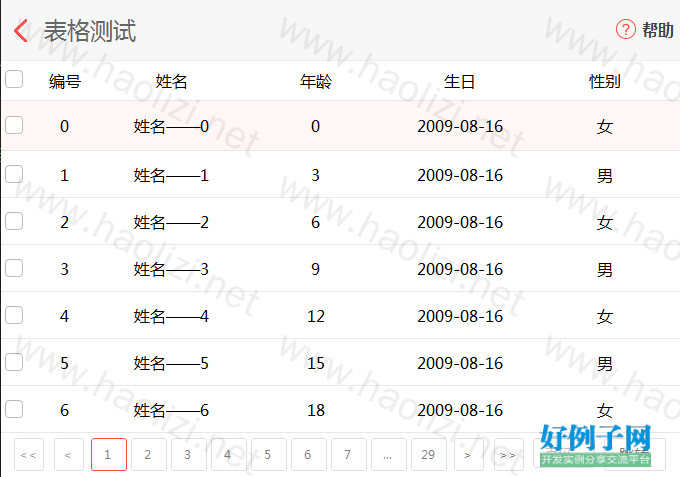
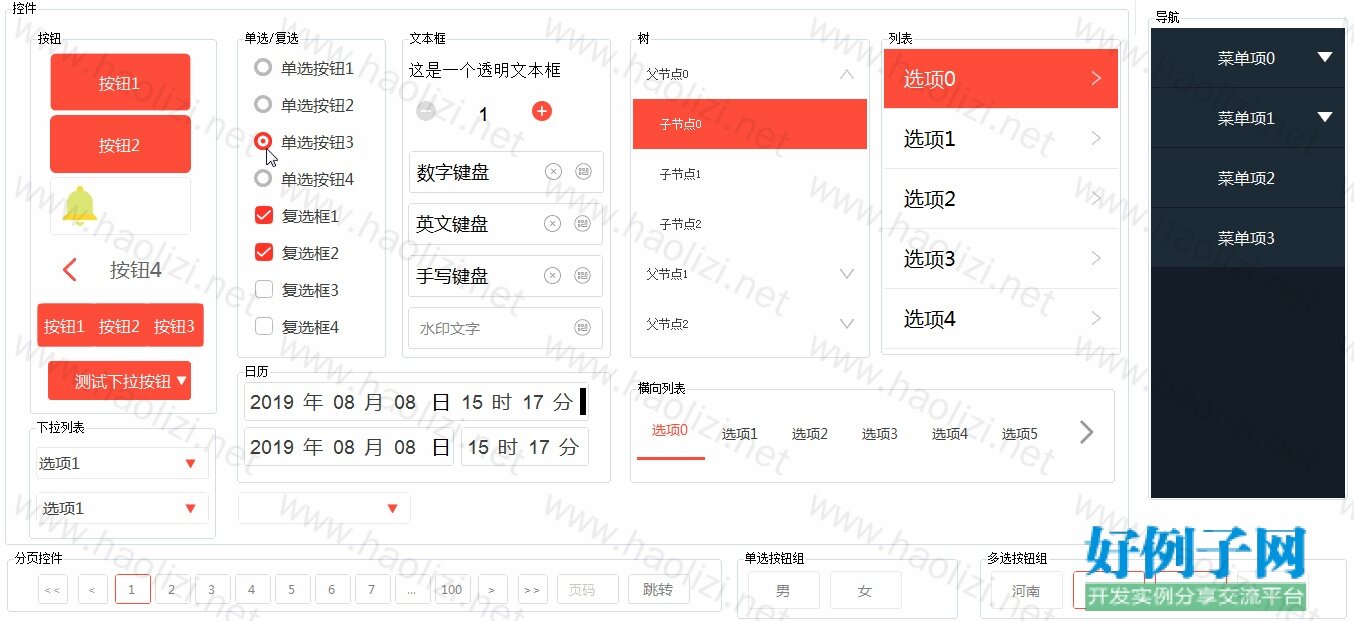
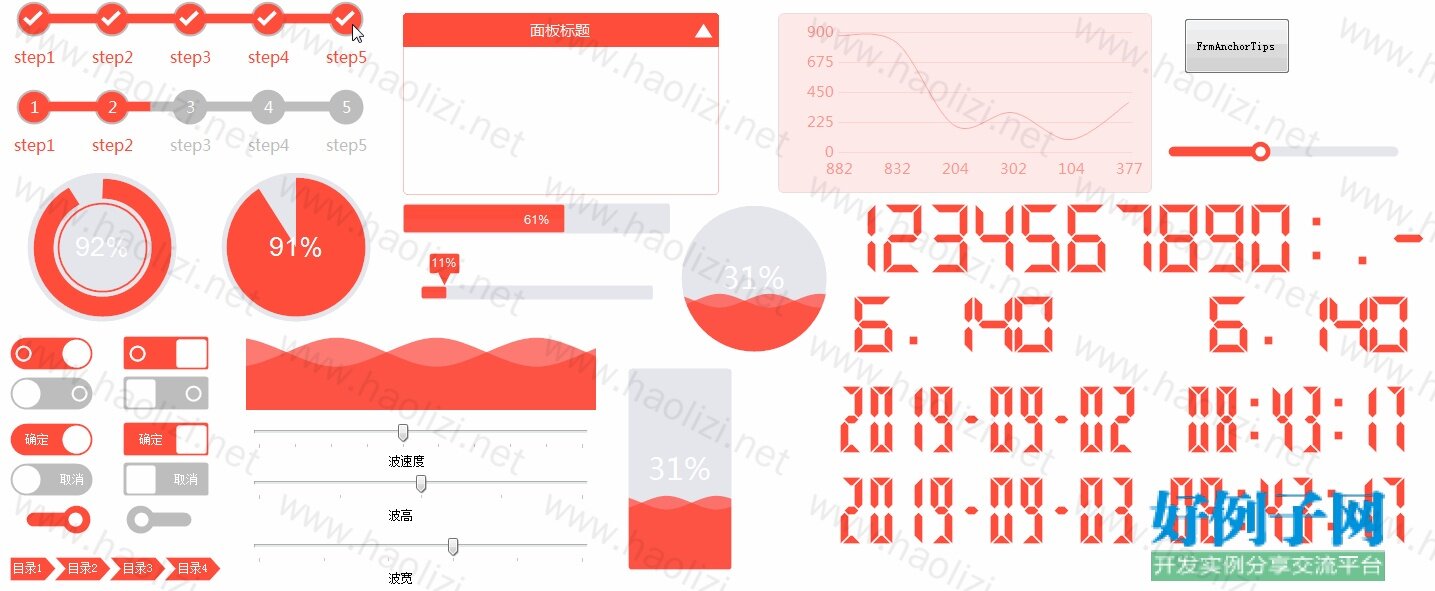
using System.Collections.Generic;
using System.ComponentModel;
using System.Drawing;
using System.Data;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace HZH_Controls.Controls
{
/// <summary>
/// Class UCBtnsGroup.
/// Implements the <see cref="System.Windows.Forms.UserControl" />
/// </summary>
/// <seealso cref="System.Windows.Forms.UserControl" />
public partial class UCBtnsGroup : UserControl
{
/// <summary>
/// 选中改变事件
/// </summary>
[Description("选中改变事件"), Category("自定义")]
public event EventHandler SelectedItemChanged;
/// <summary>
/// The m data source
/// </summary>
private Dictionary<string, string> m_dataSource = new Dictionary<string, string>();
/// <summary>
/// 数据源
/// </summary>
/// <value>The data source.</value>
[Description("数据源"), Category("自定义")]
public Dictionary<string, string> DataSource
{
get { return m_dataSource; }
set
{
m_dataSource = value;
Reload();
}
}
/// <summary>
/// The m select item
/// </summary>
private List<string> m_selectItem = new List<string>();
/// <summary>
/// 选中项
/// </summary>
/// <value>The select item.</value>
[Description("选中项"), Category("自定义")]
public List<string> SelectItem
{
get { return m_selectItem; }
set
{
m_selectItem = value;
if (m_selectItem == null)
m_selectItem = new List<string>();
SetSelected();
}
}
/// <summary>
/// The m is multiple
/// </summary>
private bool m_isMultiple = false;
/// <summary>
/// 是否多选
/// </summary>
/// <value><c>true</c> if this instance is multiple; otherwise, <c>false</c>.</value>
[Description("是否多选"), Category("自定义")]
public bool IsMultiple
{
get { return m_isMultiple; }
set { m_isMultiple = value; }
}
/// <summary>
/// Initializes a new instance of the <see cref="UCBtnsGroup" /> class.
/// </summary>
public UCBtnsGroup()
{
InitializeComponent();
}
/// <summary>
/// Reloads this instance.
/// </summary>
private void Reload()
{
try
{
ControlHelper.FreezeControl(flowLayoutPanel1, true);
this.flowLayoutPanel1.Controls.Clear();
if (DataSource != null)
{
foreach (var item in DataSource)
{
UCBtnExt btn = new UCBtnExt();
btn.BackColor = System.Drawing.Color.Transparent;
btn.BtnBackColor = System.Drawing.Color.White;
btn.BtnFont = new System.Drawing.Font("微软雅黑", 10F);
btn.BtnForeColor = System.Drawing.Color.Gray;
btn.BtnText = item.Value;
btn.ConerRadius = 5;
btn.Cursor = System.Windows.Forms.Cursors.Hand;
btn.FillColor = System.Drawing.Color.White;
btn.Font = new System.Drawing.Font("微软雅黑", 15F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Pixel);
btn.IsRadius = true;
btn.IsShowRect = true;
btn.IsShowTips = false;
btn.Location = new System.Drawing.Point(5, 5);
btn.Margin = new System.Windows.Forms.Padding(5);
btn.Name = item.Key;
btn.RectColor = System.Drawing.Color.FromArgb(224, 224, 224);
btn.RectWidth = 1;
btn.Size = new System.Drawing.Size(72, 38);
btn.TabStop = false;
btn.BtnClick = btn_BtnClick;
this.flowLayoutPanel1.Controls.Add(btn);
}
}
}
finally
{
ControlHelper.FreezeControl(flowLayoutPanel1, false);
}
SetSelected();
}
/// <summary>
/// Handles the BtnClick event of the btn control.
/// </summary>
/// <param name="sender">The source of the event.</param>
/// <param name="e">The <see cref="EventArgs" /> instance containing the event data.</param>
void btn_BtnClick(object sender, EventArgs e)
{
var btn = sender as UCBtnExt;
if (m_selectItem.Contains(btn.Name))
{
btn.RectColor = System.Drawing.Color.FromArgb(224, 224, 224);
m_selectItem.Remove(btn.Name);
}
else
{
if (!m_isMultiple)
{
foreach (var item in m_selectItem)
{
var lst = this.flowLayoutPanel1.Controls.Find(item, false);
if (lst.Length == 1)
{
var _btn = lst[0] as UCBtnExt;
_btn.RectColor = System.Drawing.Color.FromArgb(224, 224, 224);
}
}
m_selectItem.Clear();
}
btn.RectColor = System.Drawing.Color.FromArgb(255, 77, 59);
m_selectItem.Add(btn.Name);
}
if (SelectedItemChanged != null)
SelectedItemChanged(this, e);
}
/// <summary>
/// Sets the selected.
/// </summary>
private void SetSelected()
{
if (m_selectItem != null && m_selectItem.Count > 0 && DataSource != null && DataSource.Count > 0)
{
try
{
ControlHelper.FreezeControl(flowLayoutPanel1, true);
if (m_isMultiple)
{
foreach (var item in m_selectItem)
{
var lst = this.flowLayoutPanel1.Controls.Find(item, false);
if (lst.Length == 1)
{
var btn = lst[0] as UCBtnExt;
btn.RectColor = System.Drawing.Color.FromArgb(255, 77, 59);
}
}
}
else
{
UCBtnExt btn = null;
foreach (var item in m_selectItem)
{
var lst = this.flowLayoutPanel1.Controls.Find(item, false);
if (lst.Length == 1)
{
btn = lst[0] as UCBtnExt;
break;
}
}
if (btn != null)
{
m_selectItem = new List<string>() { btn.Name };
btn.RectColor = System.Drawing.Color.FromArgb(255, 77, 59);
}
}
}
finally
{
ControlHelper.FreezeControl(flowLayoutPanel1, false);
}
}
}
}
}
using System.Collections.Generic;
using System.ComponentModel;
using System.Drawing;
using System.Data;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Drawing.Drawing2D;
namespace HZH_Controls.Controls
{
/// <summary>
/// Class UCStep.
/// Implements the <see cref="System.Windows.Forms.UserControl" />
/// </summary>
/// <seealso cref="System.Windows.Forms.UserControl" />
[DefaultEvent("IndexChecked")]
public partial class UCStep : UserControl
{
/// <summary>
/// Occurs when [index checked].
/// </summary>
[Description("步骤更改事件"), Category("自定义")]
public event EventHandler IndexChecked;
/// <summary>
/// The m step back color
/// </summary>
private Color m_stepBackColor = Color.FromArgb(189, 189, 189);
/// <summary>
/// 步骤背景色
/// </summary>
/// <value>The color of the step back.</value>
[Description("步骤背景色"), Category("自定义")]
public Color StepBackColor
{
get { return m_stepBackColor; }
set
{
m_stepBackColor = value;
Refresh();
}
}
/// <summary>
/// The m step fore color
/// </summary>
private Color m_stepForeColor = Color.FromArgb(255, 77, 59);
/// <summary>
/// 步骤前景色
/// </summary>
/// <value>The color of the step fore.</value>
[Description("步骤前景色"), Category("自定义")]
public Color StepForeColor
{
get { return m_stepForeColor; }
set
{
m_stepForeColor = value;
Refresh();
}
}
/// <summary>
/// The m step font color
/// </summary>
private Color m_stepFontColor = Color.White;
/// <summary>
/// 步骤文字颜色
/// </summary>
/// <value>The color of the step font.</value>
[Description("步骤文字景色"), Category("自定义")]
public Color StepFontColor
{
get { return m_stepFontColor; }
set
{
m_stepFontColor = value;
Refresh();
}
}
/// <summary>
/// The m step width
/// </summary>
private int m_stepWidth = 35;
/// <summary>
/// 步骤宽度
/// </summary>
/// <value>The width of the step.</value>
[Description("步骤宽度景色"), Category("自定义")]
public int StepWidth
{
get { return m_stepWidth; }
set
{
m_stepWidth = value;
Refresh();
}
}
/// <summary>
/// The m steps
/// </summary>
private string[] m_steps = new string[] { "step1", "step2", "step3" };
/// <summary>
/// Gets or sets the steps.
/// </summary>
/// <value>The steps.</value>
[Description("步骤"), Category("自定义")]
public string[] Steps
{
get { return m_steps; }
set
{
if (m_steps == null || m_steps.Length <= 1)
return;
m_steps = value;
Refresh();
}
}
/// <summary>
/// The m step index
/// </summary>
private int m_stepIndex = 0;
/// <summary>
/// Gets or sets the index of the step.
/// </summary>
/// <value>The index of the step.</value>
[Description("步骤位置"), Category("自定义")]
public int StepIndex
{
get { return m_stepIndex; }
set
{
if (value > Steps.Length)
return;
m_stepIndex = value;
Refresh();
if (IndexChecked != null)
{
IndexChecked(this, null);
}
}
}
/// <summary>
/// The m line width
/// </summary>
private int m_lineWidth = 2;
/// <summary>
/// Gets or sets the width of the line.
/// </summary>
/// <value>The width of the line.</value>
[Description("连接线宽度,最小2"), Category("自定义")]
public int LineWidth
{
get { return m_lineWidth; }
set
{
if (value < 2)
return;
m_lineWidth = value;
Refresh();
}
}
/// <summary>
/// The m img completed
/// </summary>
private Image m_imgCompleted = null;
/// <summary>
/// Gets or sets the img completed.
/// </summary>
/// <value>The img completed.</value>
[Description("已完成步骤图片,当不为空时,已完成步骤将不再显示数字,建议24*24大小"), Category("自定义")]
public Image ImgCompleted
{
get { return m_imgCompleted; }
set
{
m_imgCompleted = value;
Refresh();
}
}
/// <summary>
/// The m LST cache rect
/// </summary>
List<Rectangle> m_lstCacheRect = new List<Rectangle>();
/// <summary>
/// Initializes a new instance of the <see cref="UCStep" /> class.
/// </summary>
public UCStep()
{
InitializeComponent();
this.SetStyle(ControlStyles.AllPaintingInWmPaint, true);
this.SetStyle(ControlStyles.DoubleBuffer, true);
this.SetStyle(ControlStyles.ResizeRedraw, true);
this.SetStyle(ControlStyles.Selectable, true);
this.SetStyle(ControlStyles.SupportsTransparentBackColor, true);
this.SetStyle(ControlStyles.UserPaint, true);
this.MouseDown = UCStep_MouseDown;
}
/// <summary>
/// Handles the MouseDown event of the UCStep control.
/// </summary>
/// <param name="sender">The source of the event.</param>
/// <param name="e">The <see cref="MouseEventArgs" /> instance containing the event data.</param>
void UCStep_MouseDown(object sender, MouseEventArgs e)
{
var index = m_lstCacheRect.FindIndex(p => p.Contains(e.Location));
if (index >= 0)
{
StepIndex = index 1;
}
}
/// <summary>
/// 引发 <see cref="E:System.Windows.Forms.Control.Paint" /> 事件。
/// </summary>
/// <param name="e">包含事件数据的 <see cref="T:System.Windows.Forms.PaintEventArgs" />。</param>
protected override void OnPaint(PaintEventArgs e)
{
base.OnPaint(e);
var g = e.Graphics;
g.SetGDIHigh();
if (m_steps != null && m_steps.Length > 0)
{
System.Drawing.SizeF sizeFirst = g.MeasureString(m_steps[0], this.Font);
int y = (this.Height - m_stepWidth - 10 - (int)sizeFirst.Height) / 2;
if (y < 0)
y = 0;
int intTxtY = y m_stepWidth 10;
int intLeft = 0;
if (sizeFirst.Width > m_stepWidth)
{
intLeft = (int)(sizeFirst.Width - m_stepWidth) / 2 1;
}
int intRight = 0;
System.Drawing.SizeF sizeEnd = g.MeasureString(m_steps[m_steps.Length - 1], this.Font);
if (sizeEnd.Width > m_stepWidth)
{
intRight = (int)(sizeEnd.Width - m_stepWidth) / 2 1;
}
int intSplitWidth = 20;
intSplitWidth = (this.Width - m_steps.Length - (m_steps.Length * m_stepWidth) - intRight - intLeft) / (m_steps.Length - 1);
if (intSplitWidth < 20)
intSplitWidth = 20;
m_lstCacheRect = new List<Rectangle>();
for (int i = 0; i < m_steps.Length; i )
{
#region 画圆,横线
Rectangle rectEllipse = new Rectangle(new Point(intLeft i * (m_stepWidth intSplitWidth), y), new Size(m_stepWidth, m_stepWidth));
m_lstCacheRect.Add(rectEllipse);
g.FillEllipse(new SolidBrush(m_stepBackColor), rectEllipse);
if (m_stepIndex > i)
{
g.FillEllipse(new SolidBrush(m_stepForeColor), new Rectangle(new Point(intLeft i * (m_stepWidth intSplitWidth) 2, y 2), new Size(m_stepWidth - 4, m_stepWidth - 4)));
}
if (m_stepIndex > i && m_imgCompleted != null)
{
g.DrawImage(m_imgCompleted, new Rectangle(new Point((intLeft i * (m_stepWidth intSplitWidth) (m_stepWidth - 24) / 2), y (m_stepWidth - 24) / 2), new Size(24, 24)), 0, 0, m_imgCompleted.Width, m_imgCompleted.Height, GraphicsUnit.Pixel, null);
}
else
{
System.Drawing.SizeF _numSize = g.MeasureString((i 1).ToString(), this.Font);
g.DrawString((i 1).ToString(), Font, new SolidBrush(m_stepFontColor), new Point(intLeft i * (m_stepWidth intSplitWidth) (m_stepWidth - (int)_numSize.Width) / 2 1, y (m_stepWidth - (int)_numSize.Height) / 2 1));
}
#endregion
System.Drawing.SizeF sizeTxt = g.MeasureString(m_steps[i], this.Font);
g.DrawString(m_steps[i], Font, new SolidBrush(m_stepIndex > i ? m_stepForeColor : m_stepBackColor), new Point(intLeft i * (m_stepWidth intSplitWidth) (m_stepWidth - (int)sizeTxt.Width) / 2 1, intTxtY));
}
for (int i = 0; i < m_steps.Length; i )
{
if (m_stepIndex > i)
{
if (i != m_steps.Length - 1)
{
if (m_stepIndex == i 1)
{
g.DrawLine(new Pen(m_stepForeColor, m_lineWidth), new Point(intLeft i * (m_stepWidth intSplitWidth) m_stepWidth - 3, y ((m_stepWidth) / 2)), new Point(intLeft i * (m_stepWidth intSplitWidth) m_stepWidth intSplitWidth / 2, y ((m_stepWidth) / 2)));
g.DrawLine(new Pen(m_stepBackColor, m_lineWidth), new Point(intLeft i * (m_stepWidth intSplitWidth) m_stepWidth intSplitWidth / 2, y ((m_stepWidth) / 2)), new Point(intLeft (i 1) * (m_stepWidth intSplitWidth) 10, y ((m_stepWidth) / 2)));
}
else
{
g.DrawLine(new Pen(m_stepForeColor, m_lineWidth), new Point(intLeft i * (m_stepWidth intSplitWidth) m_stepWidth - 3, y ((m_stepWidth) / 2)), new Point(intLeft (i 1) * (m_stepWidth intSplitWidth) 10, y ((m_stepWidth) / 2)));
}
}
}
else
{
if (i != m_steps.Length - 1)
{
g.DrawLine(new Pen(m_stepBackColor, m_lineWidth), new Point(intLeft i * (m_stepWidth intSplitWidth) m_stepWidth - 3, y ((m_stepWidth) / 2)), new Point(intLeft (i 1) * (m_stepWidth intSplitWidth) 10, y ((m_stepWidth) / 2)));
}
}
}
}
}
}
}
好例子网口号:伸出你的我的手 — 分享!
小贴士
感谢您为本站写下的评论,您的评论对其它用户来说具有重要的参考价值,所以请认真填写。
- 类似“顶”、“沙发”之类没有营养的文字,对勤劳贡献的楼主来说是令人沮丧的反馈信息。
- 相信您也不想看到一排文字/表情墙,所以请不要反馈意义不大的重复字符,也请尽量不要纯表情的回复。
- 提问之前请再仔细看一遍楼主的说明,或许是您遗漏了。
- 请勿到处挖坑绊人、招贴广告。既占空间让人厌烦,又没人会搭理,于人于己都无利。
关于好例子网
本站旨在为广大IT学习爱好者提供一个非营利性互相学习交流分享平台。本站所有资源都可以被免费获取学习研究。本站资源来自网友分享,对搜索内容的合法性不具有预见性、识别性、控制性,仅供学习研究,请务必在下载后24小时内给予删除,不得用于其他任何用途,否则后果自负。基于互联网的特殊性,平台无法对用户传输的作品、信息、内容的权属或合法性、安全性、合规性、真实性、科学性、完整权、有效性等进行实质审查;无论平台是否已进行审查,用户均应自行承担因其传输的作品、信息、内容而可能或已经产生的侵权或权属纠纷等法律责任。本站所有资源不代表本站的观点或立场,基于网友分享,根据中国法律《信息网络传播权保护条例》第二十二与二十三条之规定,若资源存在侵权或相关问题请联系本站客服人员,点此联系我们。关于更多版权及免责申明参见 版权及免责申明
网友评论
我要评论