【实例简介】Spring 5 中的动手响应式编程
Hands-On Reactive Programming in Spring 5
【实例截图】
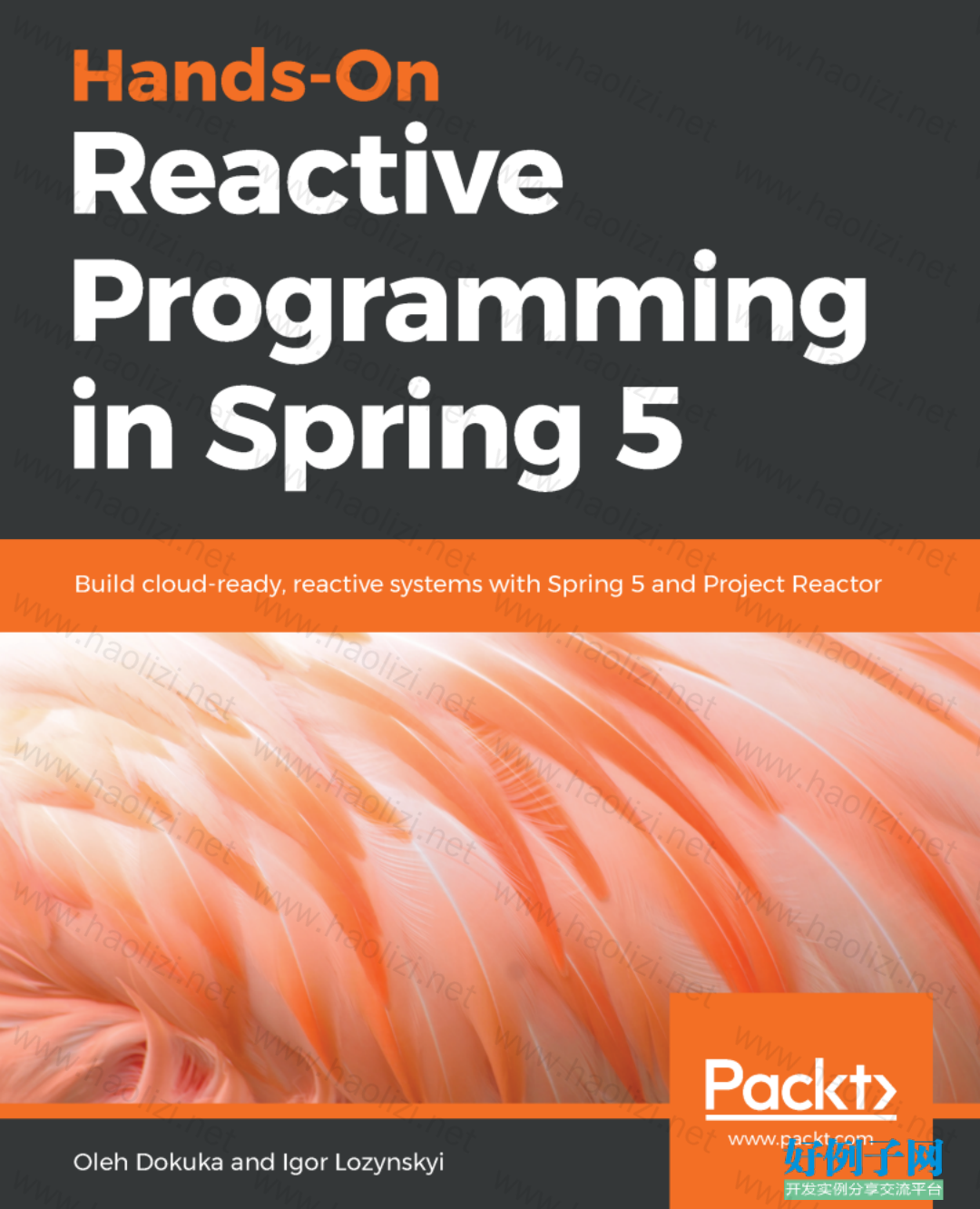
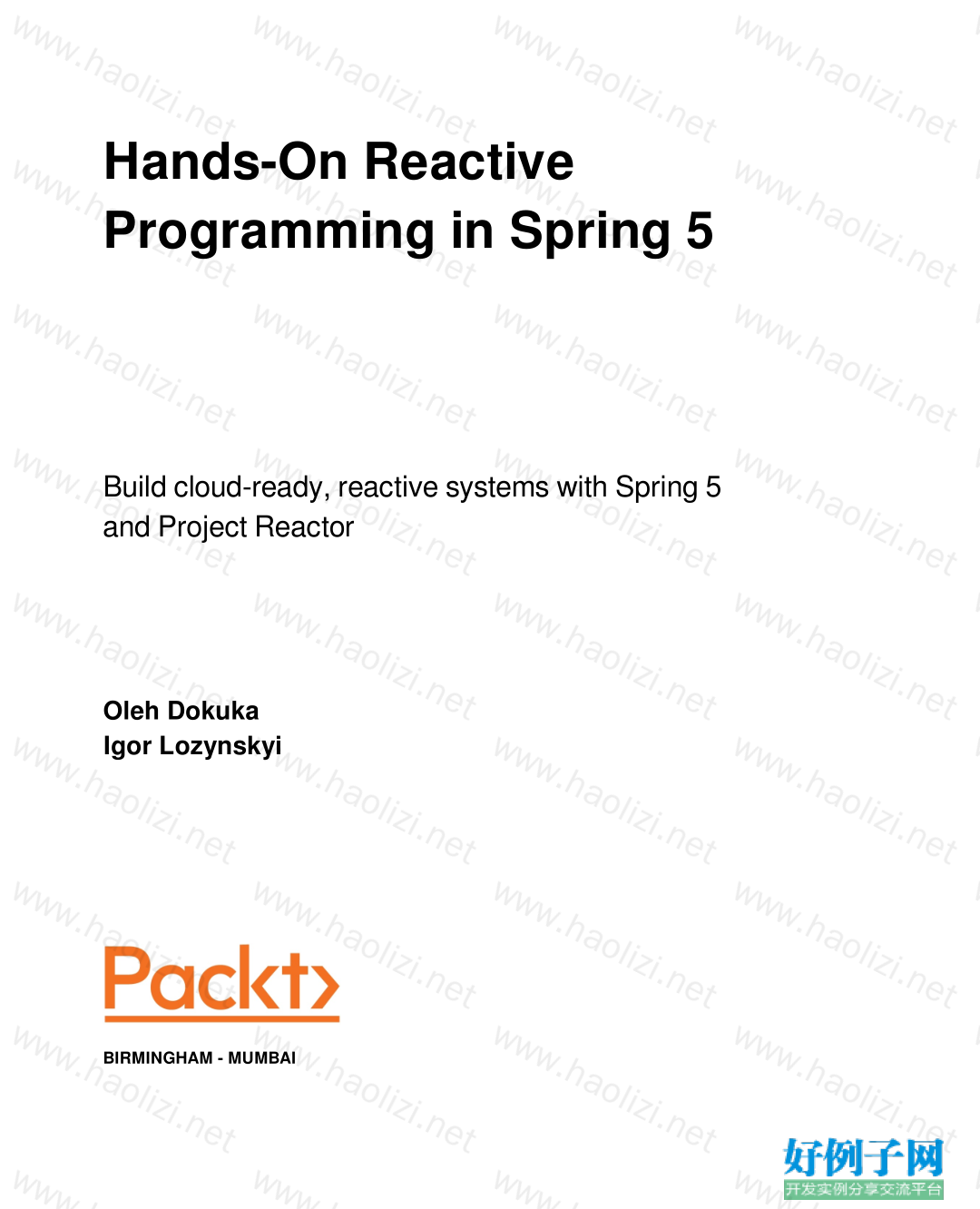

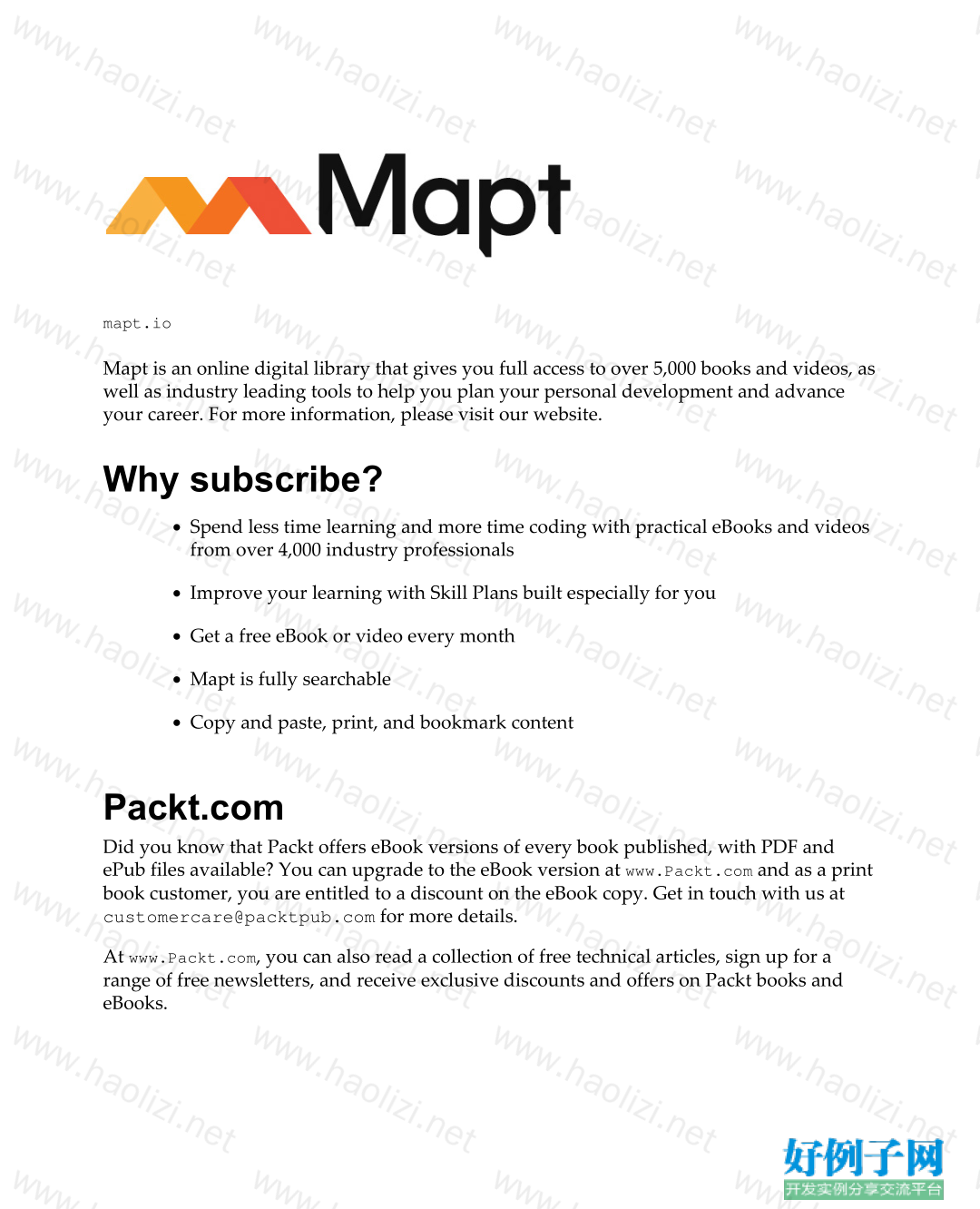
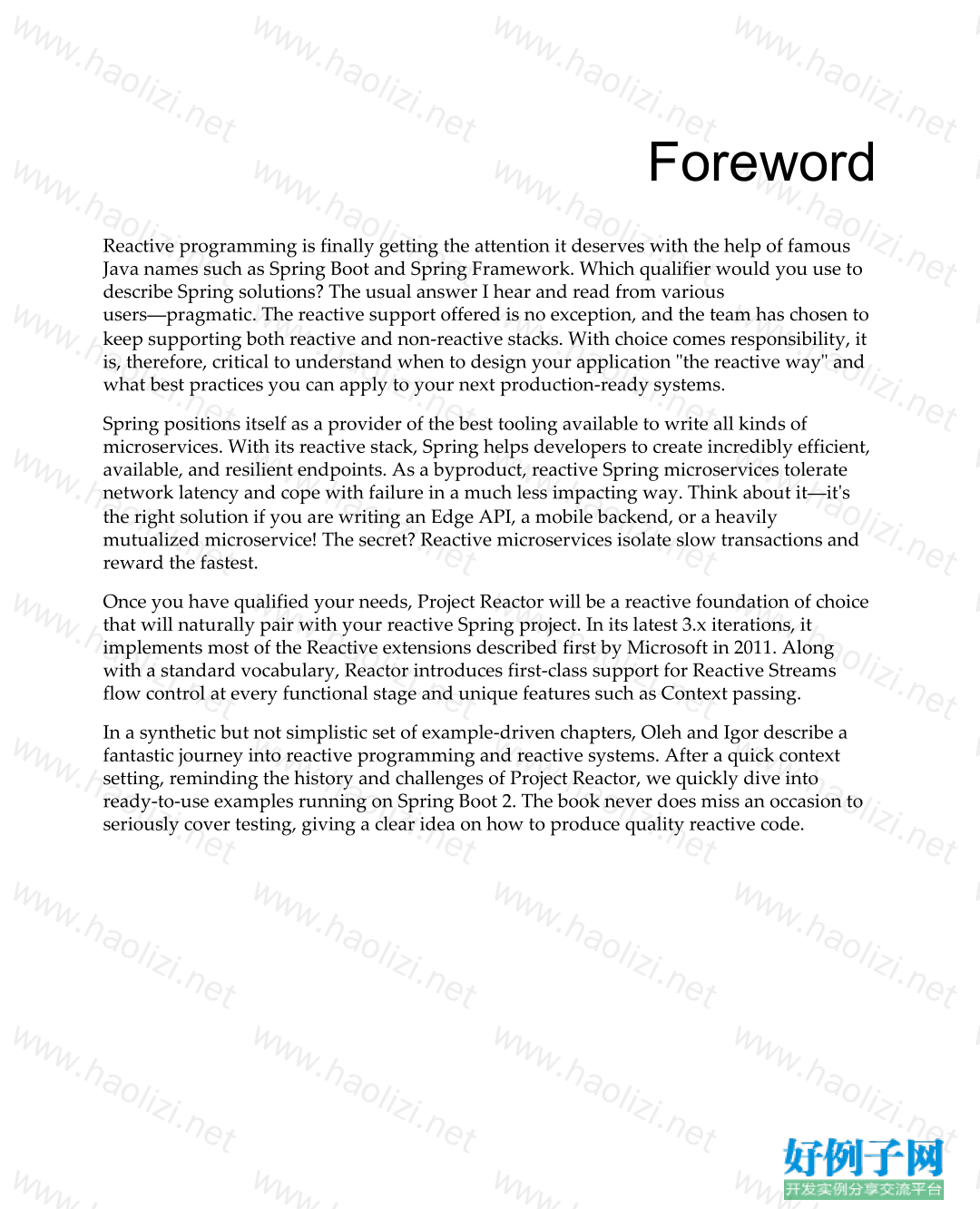
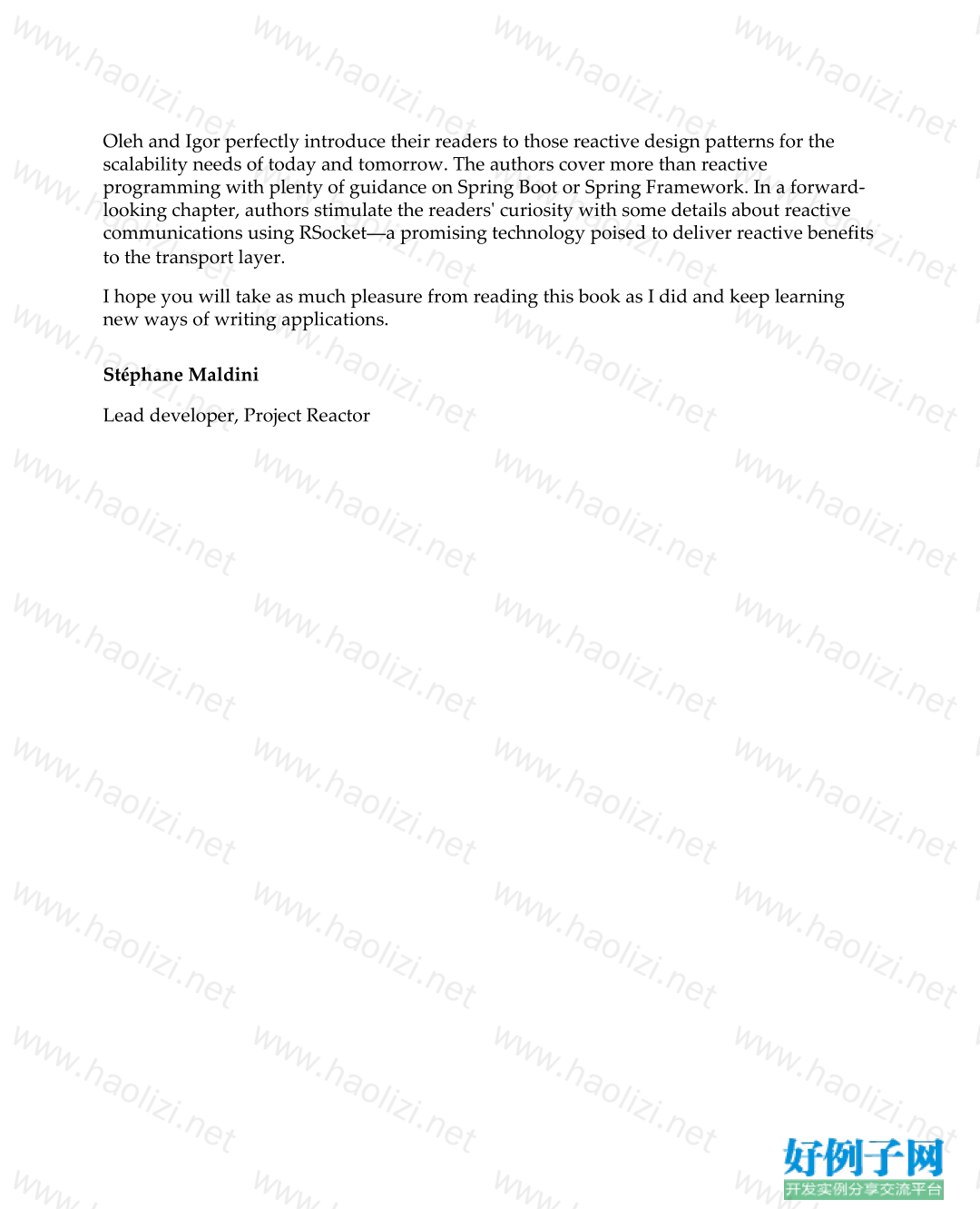

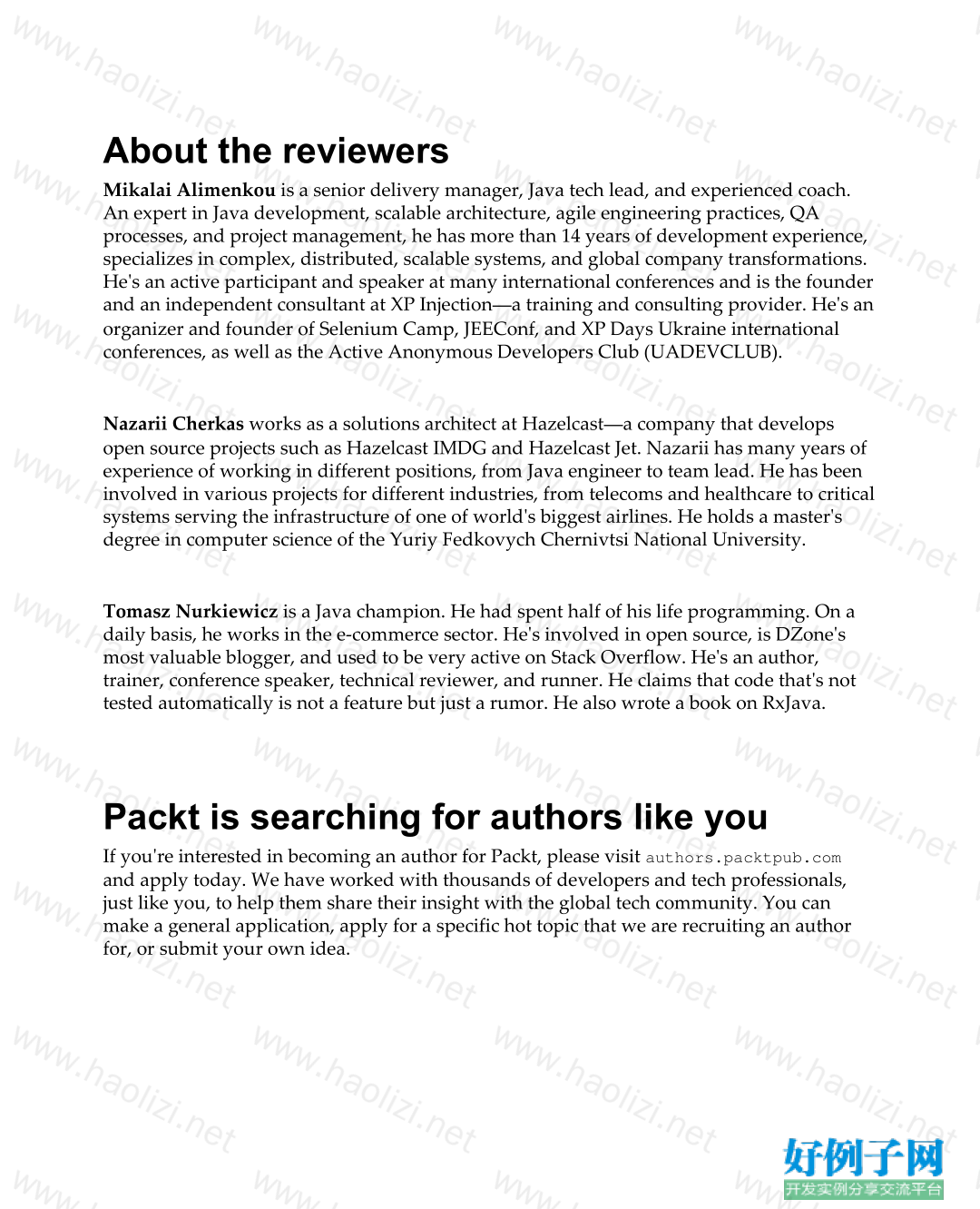
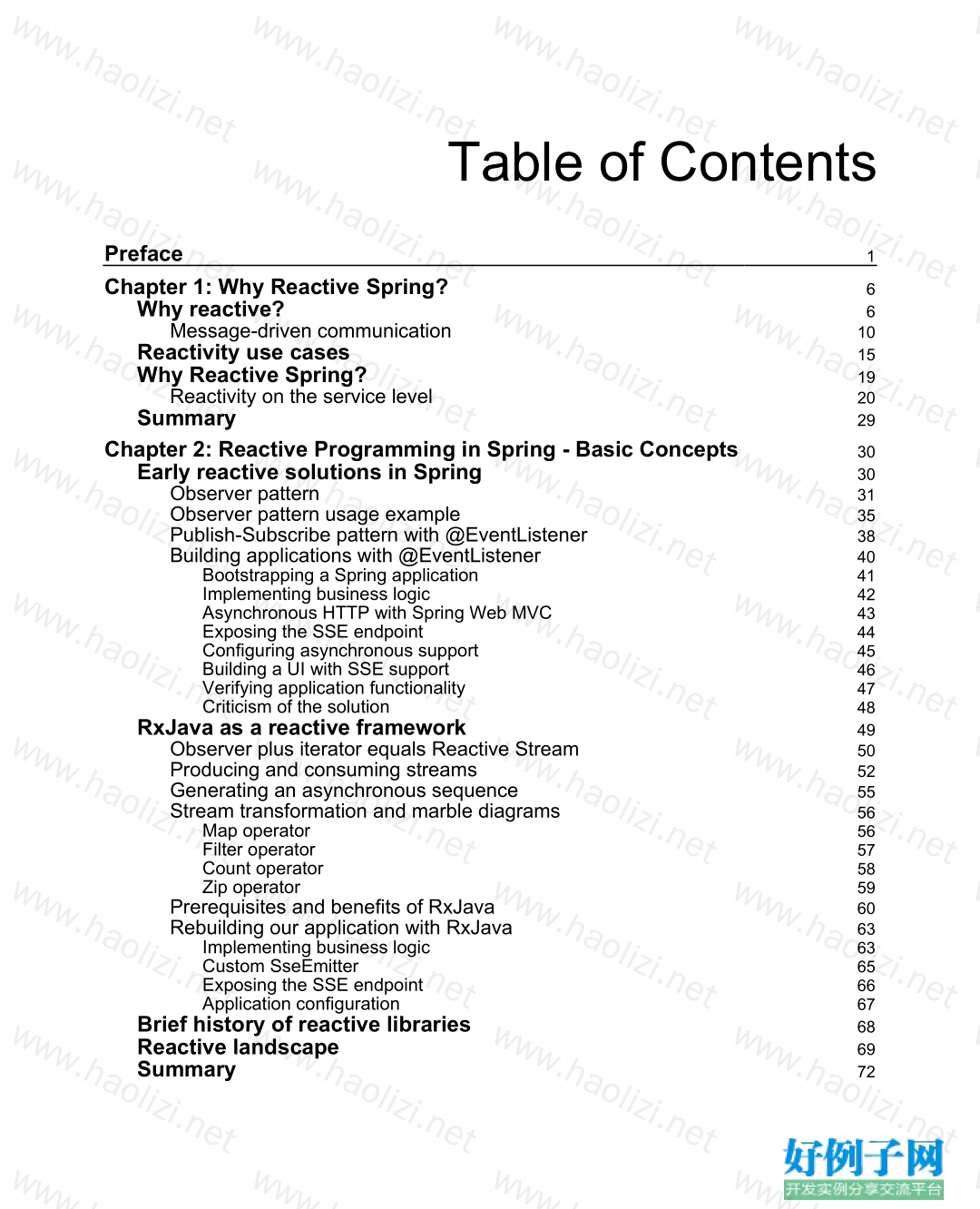
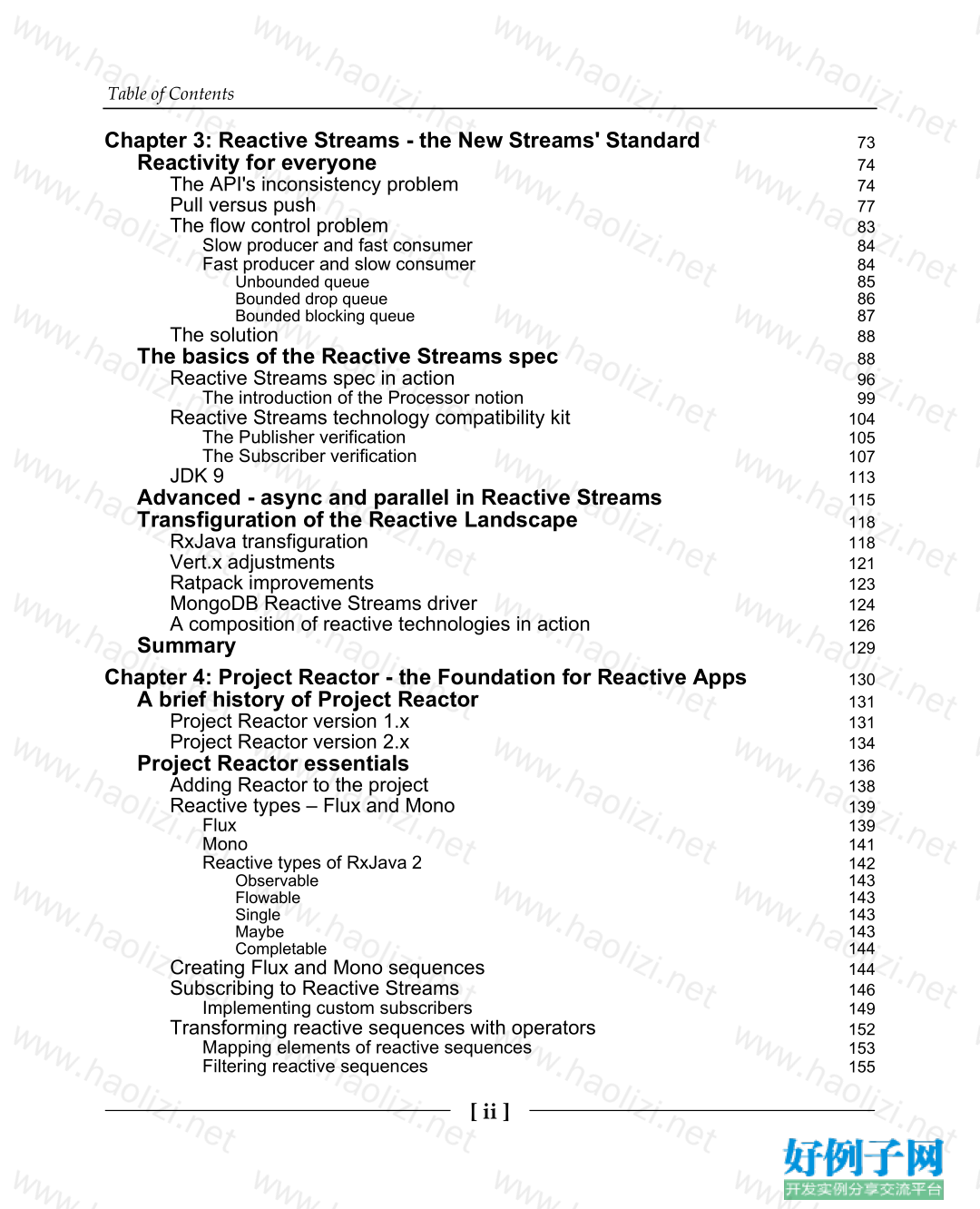
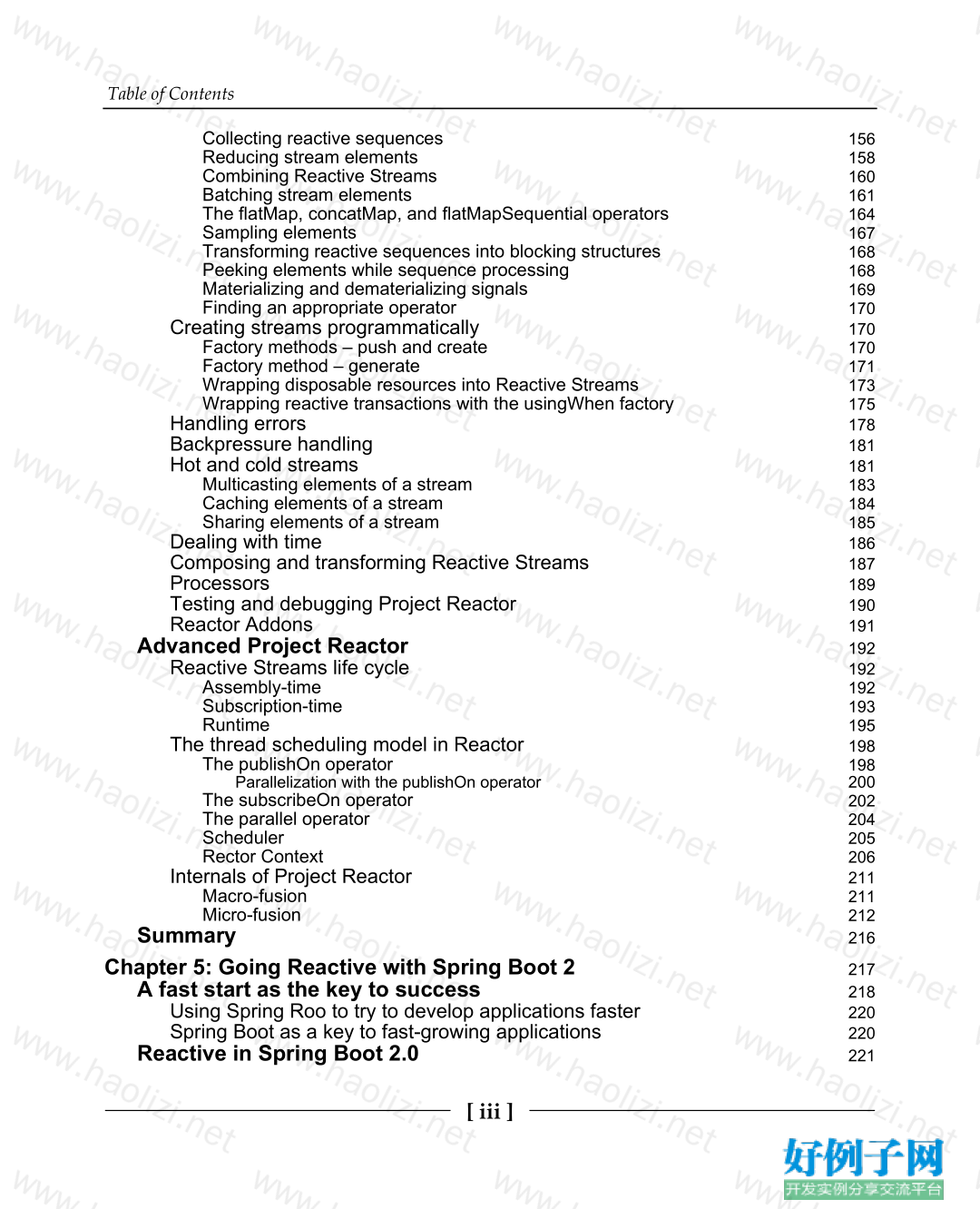
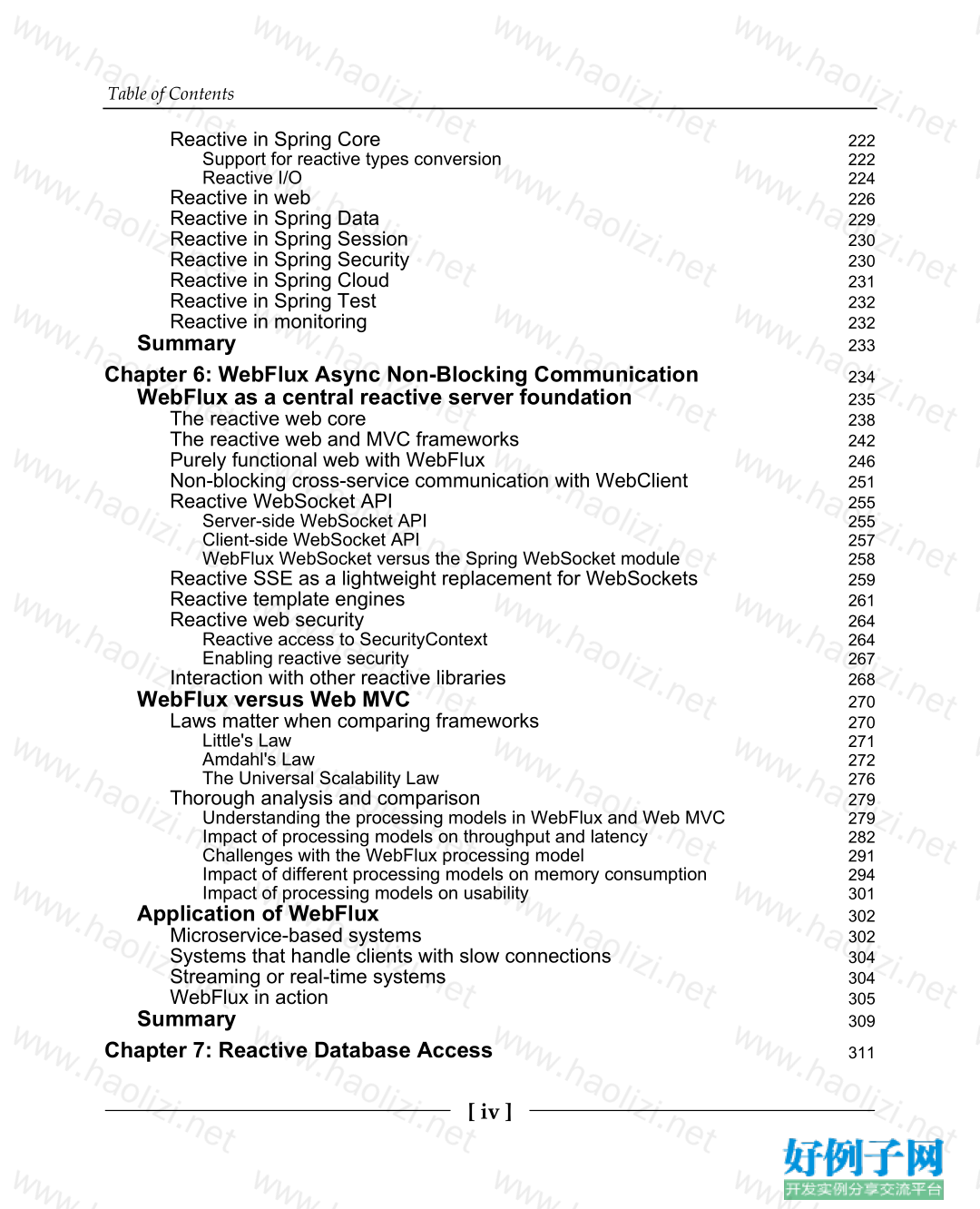
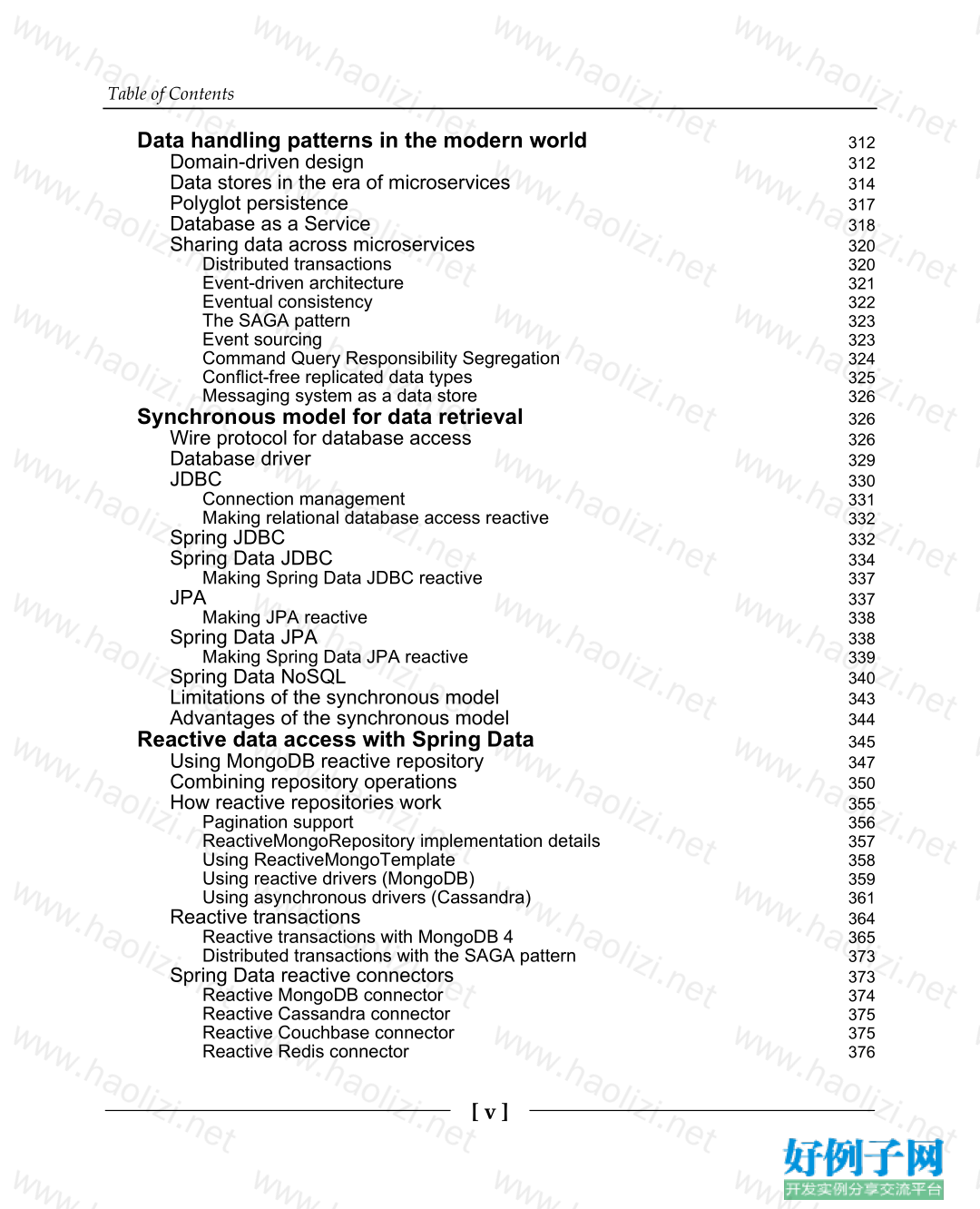
【核心代码】
Table of Contents
Preface
1
Chapter 1: Why Reactive Spring?
6
Why reactive?
6
Message-driven communication
10
Reactivity use cases
15
Why Reactive Spring?
19
Reactivity on the service level
20
Summary
29
Chapter 2: Reactive Programming in Spring - Basic Concepts
30
Early reactive solutions in Spring
30
Observer pattern
31
Observer pattern usage example
35
Publish-Subscribe pattern with @EventListener
38
Building applications with @EventListener
40
Bootstrapping a Spring application
41
Implementing business logic
42
Asynchronous HTTP with Spring Web MVC
43
Exposing the SSE endpoint
44
Configuring asynchronous support
45
Building a UI with SSE support
46
Verifying application functionality
47
Criticism of the solution
48
RxJava as a reactive framework
49
Observer plus iterator equals Reactive Stream
50
Producing and consuming streams
52
Generating an asynchronous sequence
55
Stream transformation and marble diagrams
56
Map operator
56
Filter operator
57
Count operator
58
Zip operator
59
Prerequisites and benefits of RxJava
60
Rebuilding our application with RxJava
63
Implementing business logic
63
Custom SseEmitter
65
Exposing the SSE endpoint
66
Application configuration
67
Brief history of reactive libraries
68
Reactive landscape
69
Summary
72Table of Contents
[ ii ]
Chapter 3: Reactive Streams - the New Streams' Standard
73
Reactivity for everyone
74
The API's inconsistency problem
74
Pull versus push
77
The flow control problem
83
Slow producer and fast consumer
84
Fast producer and slow consumer
84
Unbounded queue
85
Bounded drop queue
86
Bounded blocking queue
87
The solution
88
The basics of the Reactive Streams spec
88
Reactive Streams spec in action
96
The introduction of the Processor notion
99
Reactive Streams technology compatibility kit
104
The Publisher verification
105
The Subscriber verification
107
JDK 9
113
Advanced - async and parallel in Reactive Streams
115
Transfiguration of the Reactive Landscape
118
RxJava transfiguration
118
Vert.x adjustments
121
Ratpack improvements
123
MongoDB Reactive Streams driver
124
A composition of reactive technologies in action
126
Summary
129
Chapter 4: Project Reactor - the Foundation for Reactive Apps
130
A brief history of Project Reactor
131
Project Reactor version 1.x
131
Project Reactor version 2.x
134
Project Reactor essentials
136
Adding Reactor to the project
138
Reactive types – Flux and Mono
139
Flux
139
Mono
141
Reactive types of RxJava 2
142
Observable
143
Flowable
143
Single
143
Maybe
143
Completable
144
Creating Flux and Mono sequences
144
Subscribing to Reactive Streams
146
Implementing custom subscribers
149
Transforming reactive sequences with operators
152
Mapping elements of reactive sequences
153
Filtering reactive sequences
155Table of Contents
[ iii ]
Collecting reactive sequences
156
Reducing stream elements
158
Combining Reactive Streams
160
Batching stream elements
161
The flatMap, concatMap, and flatMapSequential operators
164
Sampling elements
167
Transforming reactive sequences into blocking structures
168
Peeking elements while sequence processing
168
Materializing and dematerializing signals
169
Finding an appropriate operator
170
Creating streams programmatically
170
Factory methods – push and create
170
Factory method – generate
171
Wrapping disposable resources into Reactive Streams
173
Wrapping reactive transactions with the usingWhen factory
175
Handling errors
178
Backpressure handling
181
Hot and cold streams
181
Multicasting elements of a stream
183
Caching elements of a stream
184
Sharing elements of a stream
185
Dealing with time
186
Composing and transforming Reactive Streams
187
Processors
189
Testing and debugging Project Reactor
190
Reactor Addons
191
Advanced Project Reactor
192
Reactive Streams life cycle
192
Assembly-time
192
Subscription-time
193
Runtime
195
The thread scheduling model in Reactor
198
The publishOn operator
198
Parallelization with the publishOn operator
200
The subscribeOn operator
202
The parallel operator
204
Scheduler
205
Rector Context
206
Internals of Project Reactor
211
Macro-fusion
211
Micro-fusion
212
Summary
216
Chapter 5: Going Reactive with Spring Boot 2
217
A fast start as the key to success
218
Using Spring Roo to try to develop applications faster
220
Spring Boot as a key to fast-growing applications
220
Reactive in Spring Boot 2.0
221Table of Contents
[ iv ]
Reactive in Spring Core
222
Support for reactive types conversion
222
Reactive I/O
224
Reactive in web
226
Reactive in Spring Data
229
Reactive in Spring Session
230
Reactive in Spring Security
230
Reactive in Spring Cloud
231
Reactive in Spring Test
232
Reactive in monitoring
232
Summary
233
Chapter 6: WebFlux Async Non-Blocking Communication
234
WebFlux as a central reactive server foundation
235
The reactive web core
238
The reactive web and MVC frameworks
242
Purely functional web with WebFlux
246
Non-blocking cross-service communication with WebClient
251
Reactive WebSocket API
255
Server-side WebSocket API
255
Client-side WebSocket API
257
WebFlux WebSocket versus the Spring WebSocket module
258
Reactive SSE as a lightweight replacement for WebSockets
259
Reactive template engines
261
Reactive web security
264
Reactive access to SecurityContext
264
Enabling reactive security
267
Interaction with other reactive libraries
268
WebFlux versus Web MVC
270
Laws matter when comparing frameworks
270
Little's Law
271
Amdahl's Law
272
The Universal Scalability Law
276
Thorough analysis and comparison
279
Understanding the processing models in WebFlux and Web MVC
279
Impact of processing models on throughput and latency
282
Challenges with the WebFlux processing model
291
Impact of different processing models on memory consumption
294
Impact of processing models on usability
301
Application of WebFlux
302
Microservice-based systems
302
Systems that handle clients with slow connections
304
Streaming or real-time systems
304
WebFlux in action
305
Summary
309
Chapter 7: Reactive Database Access
311Table of Contents
[ v ]
Data handling patterns in the modern world
312
Domain-driven design
312
Data stores in the era of microservices
314
Polyglot persistence
317
Database as a Service
318
Sharing data across microservices
320
Distributed transactions
320
Event-driven architecture
321
Eventual consistency
322
The SAGA pattern
323
Event sourcing
323
Command Query Responsibility Segregation
324
Conflict-free replicated data types
325
Messaging system as a data store
326
Synchronous model for data retrieval
326
Wire protocol for database access
326
Database driver
329
JDBC
330
Connection management
331
Making relational database access reactive
332
Spring JDBC
332
Spring Data JDBC
334
Making Spring Data JDBC reactive
337
JPA
337
Making JPA reactive
338
Spring Data JPA
338
Making Spring Data JPA reactive
339
Spring Data NoSQL
340
Limitations of the synchronous model
343
Advantages of the synchronous model
344
Reactive data access with Spring Data
345
Using MongoDB reactive repository
347
Combining repository operations
350
How reactive repositories work
355
Pagination support
356
ReactiveMongoRepository implementation details
357
Using ReactiveMongoTemplate
358
Using reactive drivers (MongoDB)
359
Using asynchronous drivers (Cassandra)
361
Reactive transactions
364
Reactive transactions with MongoDB 4
365
Distributed transactions with the SAGA pattern
373
Spring Data reactive connectors
373
Reactive MongoDB connector
374
Reactive Cassandra connector
375
Reactive Couchbase connector
375
Reactive Redis connector
376Table of Contents
[ vi ]
Limitations and anticipated improvements
377
Asynchronous Database Access
378
Reactive Relational Database Connectivity
380
Using R2DBC with Spring Data R2DBC
382
Transforming a synchronous repository into reactive
383
Using the rxjava2-jdbc library
384
Wrapping a synchronous CrudRepository
386
Reactive Spring Data in action
390
Summary
394
Chapter 8: Scaling Up with Cloud Streams
396
Message brokers as the key to message-driven systems
397
Server-side load balancing
397
Client-side load balancing with Spring Cloud and Ribbon
400
Message brokers as an elastic, reliable layer for message transferring
406
The market of message brokers
411
Spring Cloud Streams as a bridge to Spring Ecosystem
412
Reactive programming in the cloud
423
Spring Cloud Data Flow
423
The finest-grained application with Spring Cloud Function
426
Spring Cloud – function as a part of a data flow
434
RSocket for low-latency, reactive message passing
439
RSocket versus Reactor-Netty
440
RSocket in Java
444
RSocket versus gRPC
448
RSocket in Spring Framework
450
RSocket in other frameworks
452
The ScaleCube Project
453
The Proteus Project
453
Summarizing RSocket
454
Summary
454
Chapter 9: Testing the Reactive Application
456
Why are reactive streams hard to test?
457
Testing reactive streams with StepVerifier
457
Essentials of StepVerifier
458
Advanced testing with StepVerifier
461
Dealing with virtual time
463
Verifying reactive context
466
Testing WebFlux
467
Testing Controllers with WebTestClient
467
Testing WebSocket
473
Summary
476
Chapter 10: And, Finally, Release It!
478
The importance of DevOps-friendly apps
478Table of Contents
[ vii ]
Monitoring the Reactive Spring application
482
Spring Boot Actuator
482
Adding an actuator to the project
482
Service info endpoint
483
Health information endpoint
485
Metrics endpoint
488
Loggers management endpoint
490
Other valuable endpoints
491
Writing custom actuator endpoints
492
Securing actuator endpoints
494
Micrometer
495
Default Spring Boot metrics
496
Monitoring Reactive Streams
497
Monitoring reactor flows
497
Monitoring reactor schedulers
498
Adding custom Micrometer meters
501
Distributed tracing with Spring Boot Sleuth
502
Pretty UI with Spring Boot Admin 2.x
503
Deploying to the cloud
506
Deploying to Amazon Web Services
509
Deploying to the Google Kubernetes Engine
510
Deploying to Pivotal Cloud Foundry
510
Discovering RabbitMQ in PCF
512
Discovering MongoDB in PCF
513
Configurationless deployment with Spring Cloud Data Flow for PCF
515
Knative for FaaS over Kubernetes and Istio
515
Bits of advice for successful application deployment
516
Summary
517
Other Books You May Enjoy
519
Index
522
网友评论
我要评论