【实例简介】面向 Android 开发人员的 Manning RxJava
Manning RxJava for Android Developers
【实例截图】
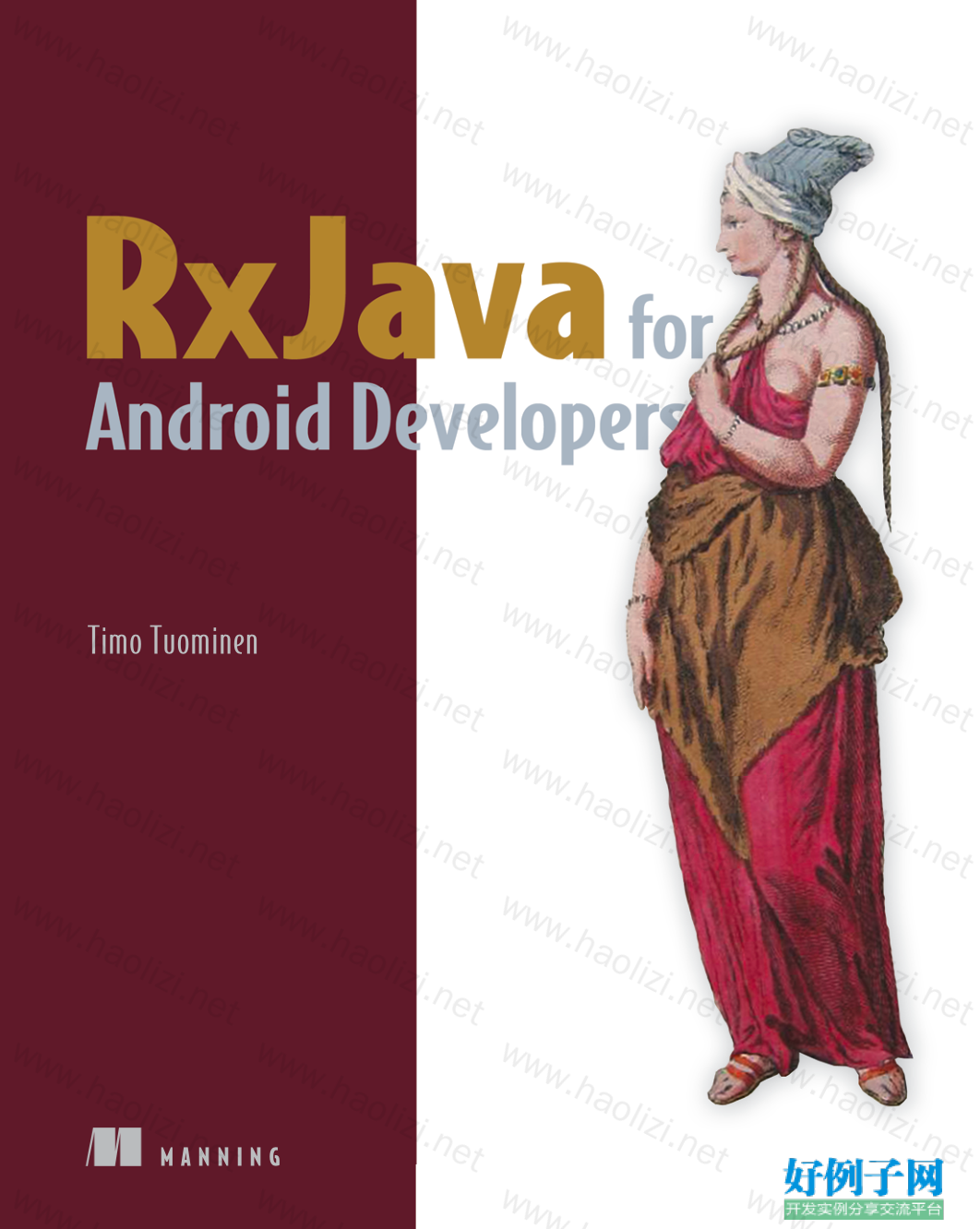



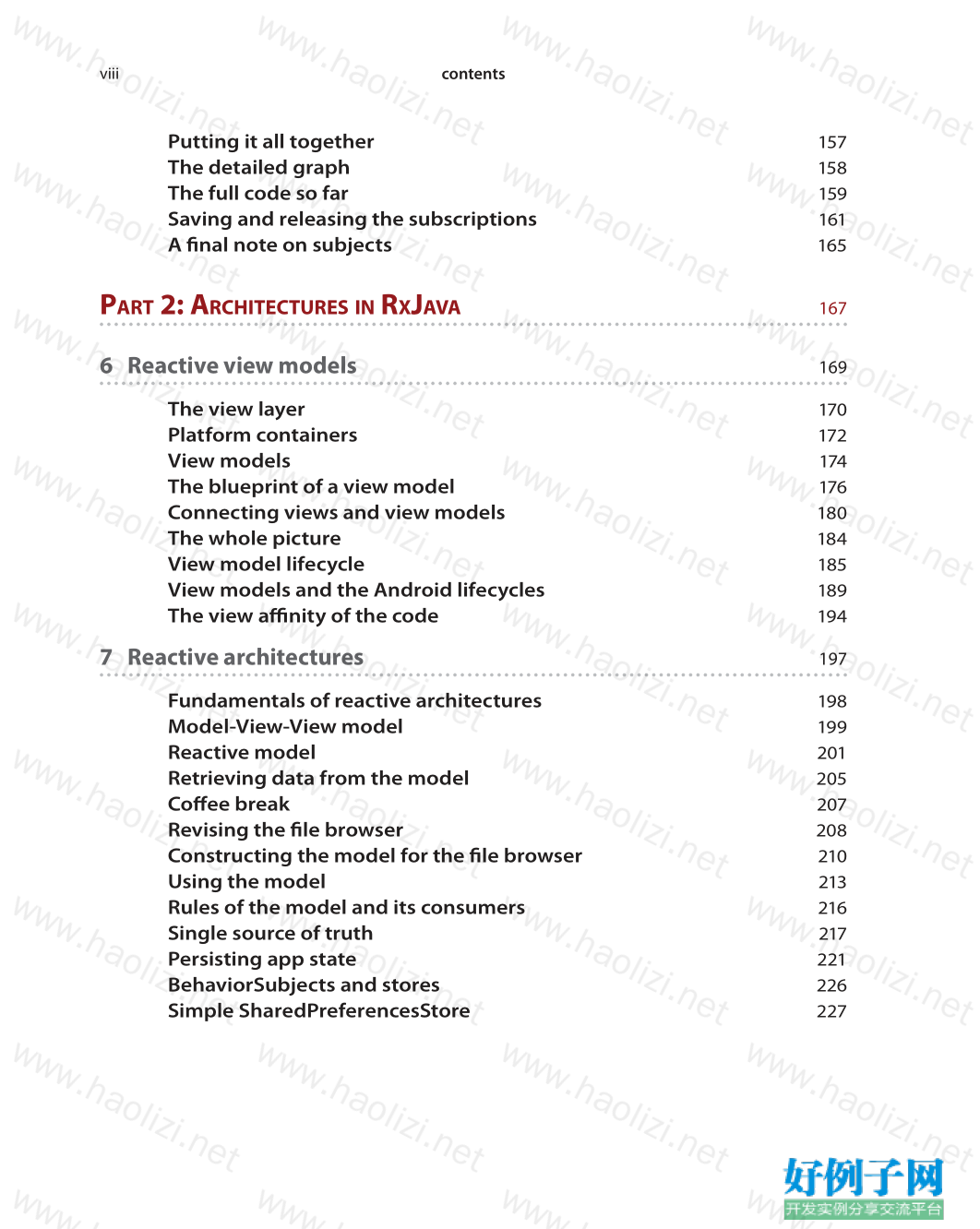
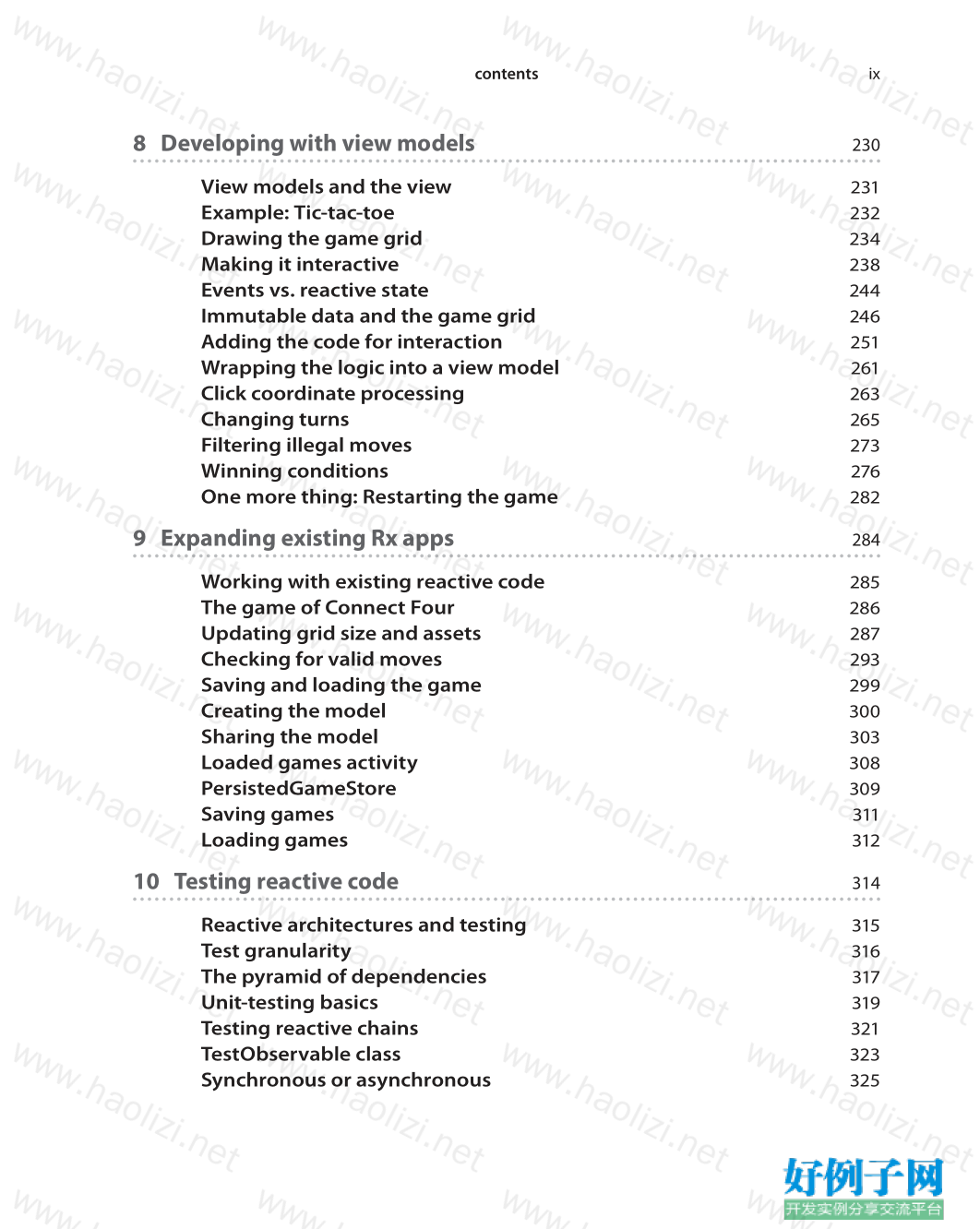
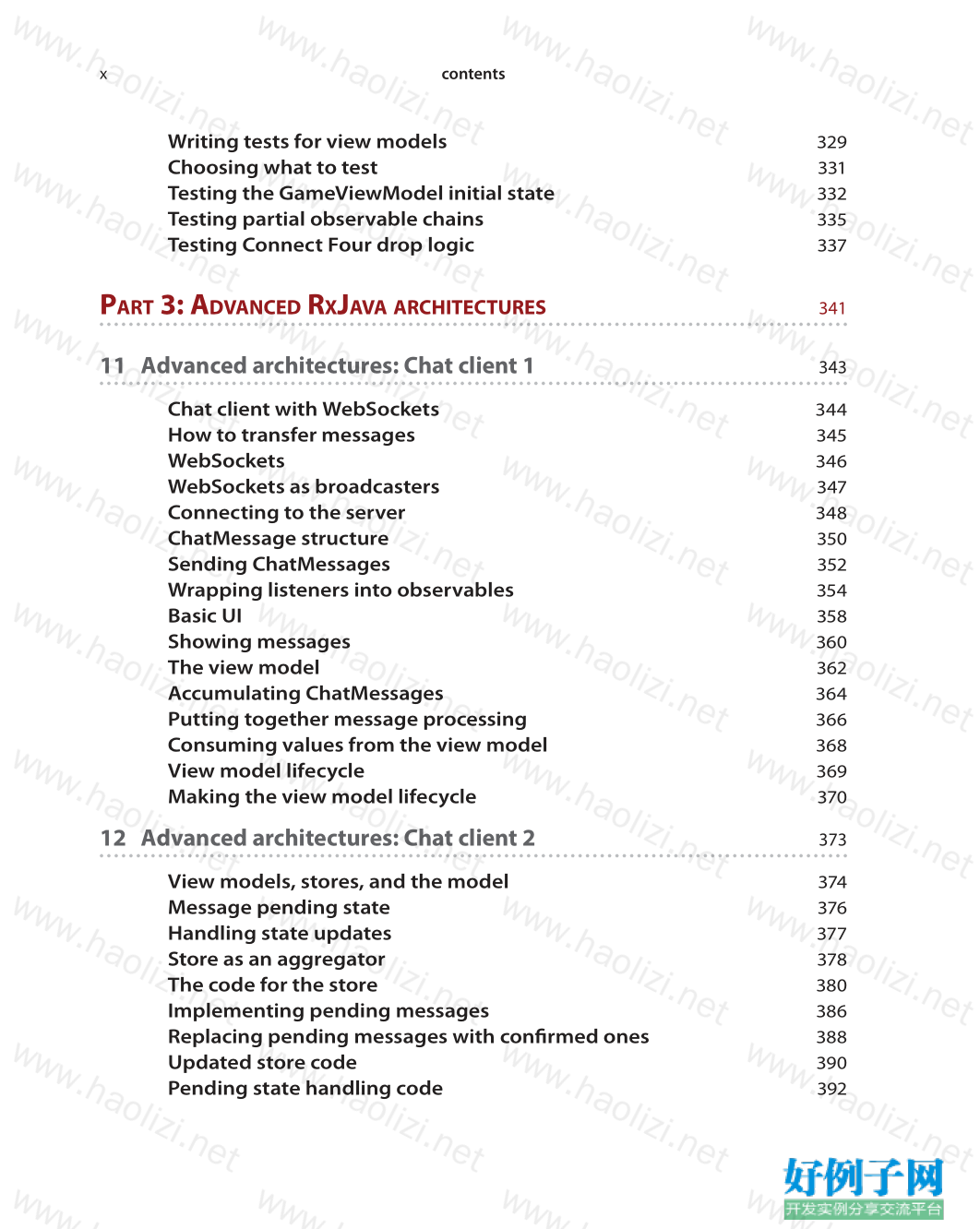
【核心代码】
contents
preface
xiii
acknowledgments
xv
about this book
xvi
Part 1: Core reactive programming
1
1 Introduction to reactive programming
3
Perhaps you picked up this book because...
4
Don’t read this book if...
5
OOP, Rx, FP, and FRP
6
Benefits of Rx
7
What you code is what you get
8
The reactive landscape
10
What do you need to know before you start?
12
About this book
13
RxJava 2 and Android
14
Setting up the Android environment
15
Java 8 lambdas
16
Deep dive into Rx: Live search
18
Project setup
19
Text input as an emitter of data
20
The publish-subscribe pattern
21
Text input as an observable
22
Filtering observables
23
Bending time
25vi
contents
Bending time to your benefit
26
Debounce: The bus stop operator
27
Putting your code into Android
30
Principles of reactive programming
32
Events and marble diagrams
33
2 Networking with observables
35
RxJava and event streams
36
Subscribers
37
RxJava 2 observable types
38
Subscribing to and converting different observables
39
What happens when you make a normal network request?
40
What happens when you make a network request with
an observable?
41
Network request as an observable
42
Example: An RSS feed aggregator
43
The feed structure
44
Getting the data
45
The combineLatest operator
46
The Rx code so far
47
Asynchronous data processing chains
49
Putting the list in order
51
The map operator
52
Brief introduction to immutability
55
Error handling
59
Adding more feeds to the client
62
3 Building data processing chains
65
Different roles of observables
66
Events vs. reactive state
68
Internal state of an observable
70
Arrow diagrams and different observable types
71
Example: Credit card validation form
72
First step: Expiration date
76
Credit card number type and checksum
78
CVC code validation
82
Putting it all together
84vii
contents
The abstraction level of reactive programming
87
How RxJava works
89
4 Connecting the user interface with networking
91
Subscriptions explained
92
Terminating subscriptions
94
RxJava 2 concepts and subscription management
96
Advanced Rx chains example: Flickr search client
98
Setting up the Flickr client project
99
Overview of the search chain
101
Step 1: A simple hardcoded search
102
Making it click
104
Implementing the reactive chain
108
How switchMap works
110
Getting thumbnail information
112
Step 1: Expand the list into an observable
113
Step 2: Apply operations to each item individually
118
Step 3: Collect results
119
The complete solution
120
Adding a username from another API
122
5 Advanced RxJava
125
Observables and subjects in detail
126
Example: File browser
127
User flow of the File Browser app
128
Getting the file listing for a directory
130
Threading basics
134
Threading in functional programming
136
Changing the thread by using getFileListingObservable
138
Making the file listing dynamic
142
Making the list click
144
Different types of subjects
146
Using a subject as the FileObservable
148
Adding buttons for Previous and Root
151
Expanded graph for Previous and Root
152
Improved version with cleaner observables
154
The Previous button
156viii
contents
Putting it all together
157
The detailed graph
158
The full code so far
159
Saving and releasing the subscriptions
161
A final note on subjects
165
Part 2: Architectures in RxJava
167
6 Reactive view models
169
The view layer
170
Platform containers
172
View models
174
The blueprint of a view model
176
Connecting views and view models
180
The whole picture
184
View model lifecycle
185
View models and the Android lifecycles
189
The view affinity of the code
194
7 Reactive architectures
197
Fundamentals of reactive architectures
198
Model-View-View model
199
Reactive model
201
Retrieving data from the model
205
Coffee break
207
Revising the file browser
208
Constructing the model for the file browser
210
Using the model
213
Rules of the model and its consumers
216
Single source of truth
217
Persisting app state
221
BehaviorSubjects and stores
226
Simple SharedPreferencesStore
227ix
contents
8 Developing with view models
230
View models and the view
231
Example: Tic-tac-toe
232
Drawing the game grid
234
Making it interactive
238
Events vs. reactive state
244
Immutable data and the game grid
246
Adding the code for interaction
251
Wrapping the logic into a view model
261
Click coordinate processing
263
Changing turns
265
Filtering illegal moves
273
Winning conditions
276
One more thing: Restarting the game
282
9 Expanding existing Rx apps
284
Working with existing reactive code
285
The game of Connect Four
286
Updating grid size and assets
287
Checking for valid moves
293
Saving and loading the game
299
Creating the model
300
Sharing the model
303
Loaded games activity
308
PersistedGameStore
309
Saving games
311
Loading games
312
10 Testing reactive code
314
Reactive architectures and testing
315
Test granularity
316
The pyramid of dependencies
317
Unit-testing basics
319
Testing reactive chains
321
TestObservable class
323
Synchronous or asynchronous
325x
Writing tests for view models
329
Choosing what to test
331
Testing the GameViewModel initial state
332
Testing partial observable chains
335
Testing Connect Four drop logic
337
Part 3: Advanced RxJava architectures
341
11 Advanced architectures: Chat client 1
343
Chat client with WebSockets
344
How to transfer messages
345
WebSockets
346
WebSockets as broadcasters
347
Connecting to the server
348
ChatMessage structure
350
Sending ChatMessages
352
Wrapping listeners into observables
354
Basic UI
358
Showing messages
360
The view model
362
Accumulating ChatMessages
364
Putting together message processing
366
Consuming values from the view model
368
View model lifecycle
369
Making the view model lifecycle
370
12 Advanced architectures: Chat client 2
373
View models, stores, and the model
374
Message pending state
376
Handling state updates
377
Store as an aggregator
378
The code for the store
380
Implementing pending messages
386
Replacing pending messages with confirmed ones
388
Updated store code
390
Pending state handling code
392
contentsxi
The model
396
The code for the model
398
Model lifecycle
400
Singleton modules
402
Android tools for production use
404
13 Transitions with Rx
407
Transitioning between states
408
How it should have been
410
Animation progress using one number
412
Reactive parametrization
413
Example: The fan widget
414
Setting the transformations for the child views
416
Animating with RxJava and Android
421
Code changes to FanView
422
The view model
424
View model logic
426
Animating parametrized values in view models
428
animateTo operator
430
animateTo operator on Android
432
Adding a dimmed background
434
14 Making a maps client
437
Maps example
438
Getting started with map tiles
440
Making an initial view model
444
Calculating tiles based on zoom level
446
Using the offset to move tiles
450
Dragging the map
452
The code so far
455
Viewport and hiding tiles
456
Loading map tiles
459
Adding zoom-level controls
462
Adding support for map coordinates
464
Appendix: Tutorial for developing on Android
469
index
483
网友评论
我要评论