实例介绍
【实例截图】
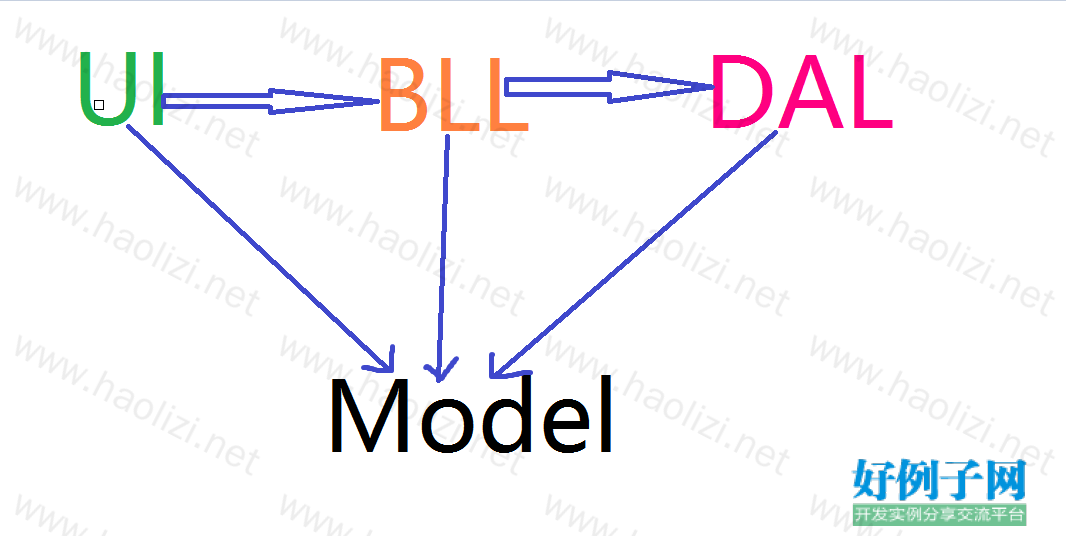
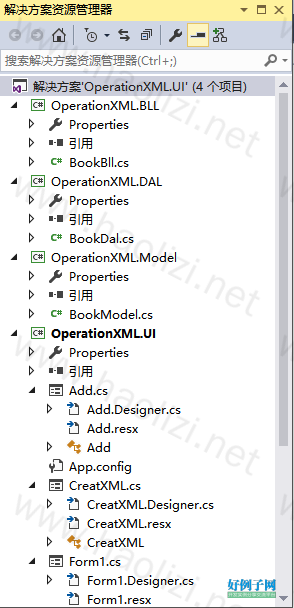
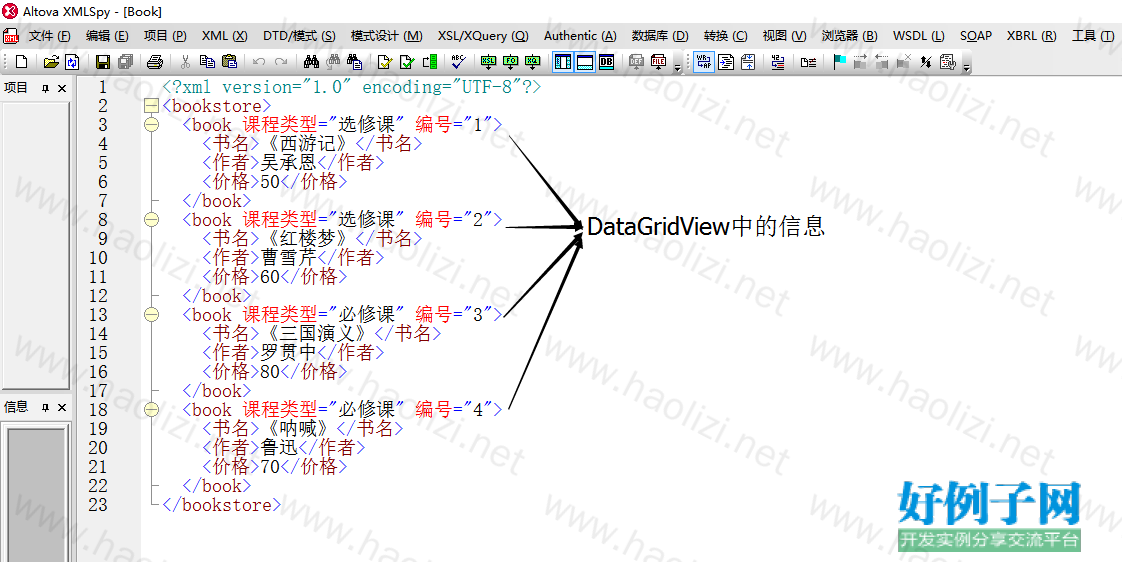

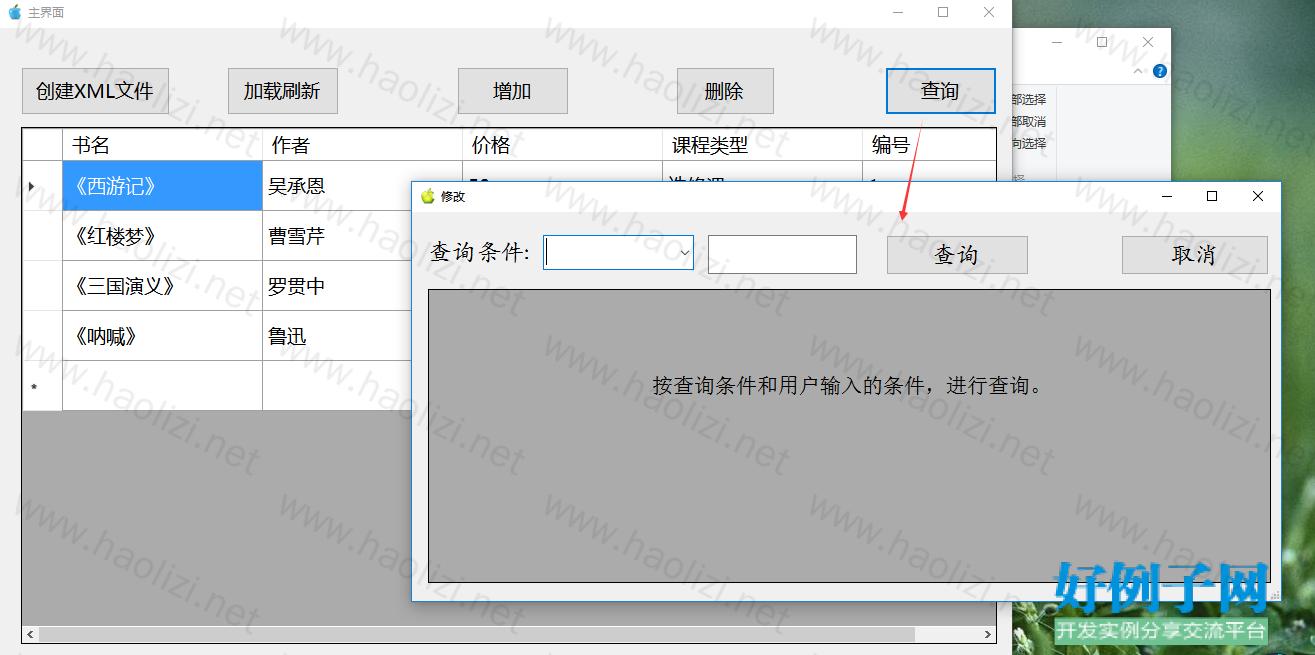
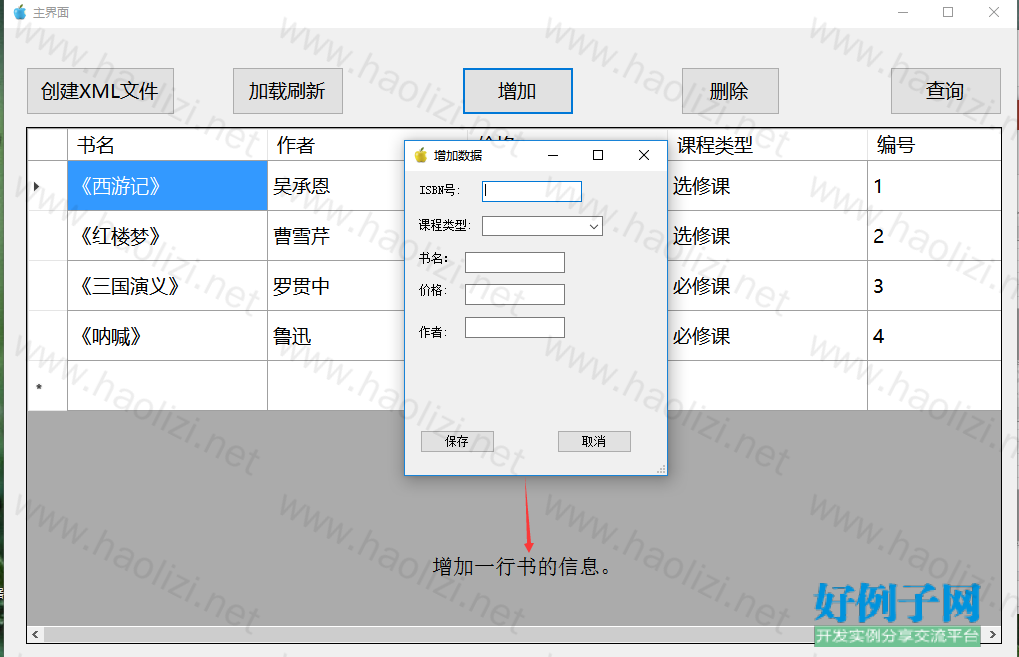
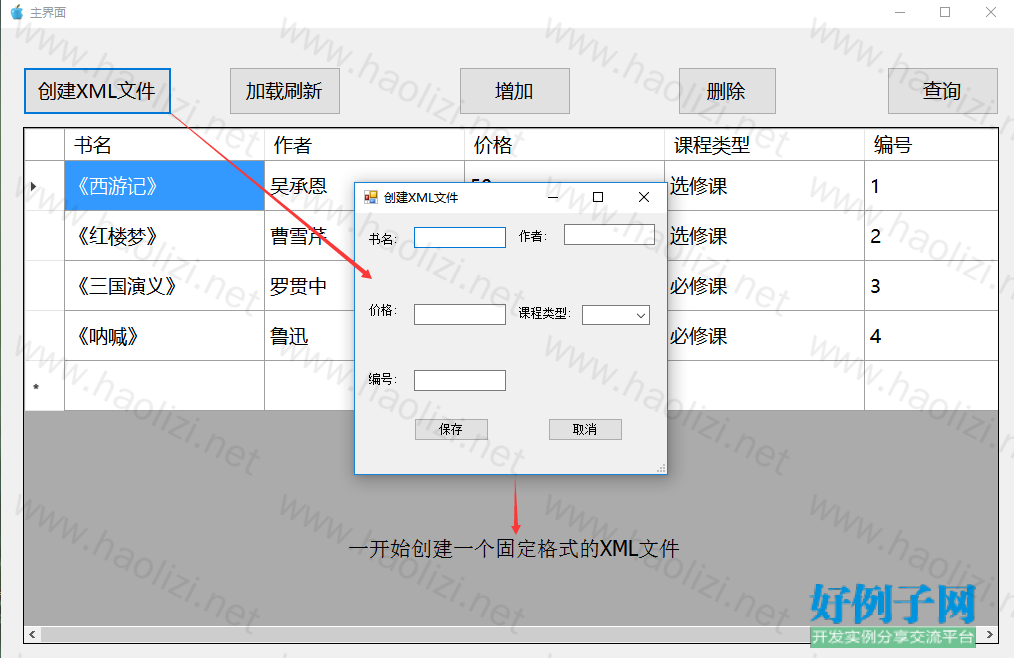
【核心代码】
Model 层代码:
namespace OperationXML.Model
{
public class BookModel
{
private string _bookType;
public string BookType
{
get { return _bookType; }
set { _bookType = value; }
}
private int _bookID;
public int BookID
{
get { return _bookID; }
set { _bookID = value; }
}
private string _bookName;
public string BookName
{
get { return _bookName; }
set { _bookName = value; }
}
private string _bookAuthor;
public string BookAuthor
{
get { return _bookAuthor; }
set { _bookAuthor = value; }
}
private string _bookPrice;
public string BookPrice
{
get { return _bookPrice; }
set { _bookPrice = value; }
}
}
}
DAL(数据访问层)代码:
using OperationXML.Model;
using System;
using System.Collections.Generic;
using System.Data;
using System.Linq;
using System.Xml;
using System.Xml.Linq;
namespace OperationXML.DAL
{
public class BookDal
{
static string strPath = "Book.xml";
public void CreatBookInfo(BookModel _bookmodel)
{
XmlDocument doc = new XmlDocument(); //实例化XmlDocument对象
XmlDeclaration dec = doc.CreateXmlDeclaration("1.0", "UTF-8", null); //XML头
doc.AppendChild(dec);
XmlElement root = doc.CreateElement("bookstore"); //根节点
doc.AppendChild(root);
XmlElement xml0 = doc.CreateElement("book"); //子根节点
xml0.SetAttribute("课程类型", _bookmodel.BookType); //设置属性。由UI端写入数据。
xml0.SetAttribute("编号", Convert.ToString(_bookmodel.BookID));
XmlElement xml1 = doc.CreateElement("书名");//创建一个书名节点
xml1.InnerText = _bookmodel.BookName;
xml0.AppendChild(xml1);//添加
XmlElement xml2 = doc.CreateElement("作者");//创建作者节点
xml2.InnerText = _bookmodel.BookAuthor;
xml0.AppendChild(xml2);//添加
XmlElement xml3 = doc.CreateElement("价格");//创建一个价格节点
xml3.InnerText = _bookmodel.BookPrice;
xml0.AppendChild(xml3);//添加
root.AppendChild(xml0);
doc.Save("Book.xml");
} //创建
public DataSet LoadBookInfo()
{
DataSet ds = new DataSet();
ds.ReadXml(strPath);
return ds;
} //加载到datagridview中
public void AddBookInfo(BookModel _bookmodel)
{
XmlDocument doc = new XmlDocument();
doc.Load("Book.xml");
XmlNode root = doc.SelectSingleNode("bookstore");
XmlElement xelKey = doc.CreateElement("book");
XmlAttribute a = doc.CreateAttribute("课程类型");
a.InnerText = _bookmodel.BookType;
xelKey.SetAttributeNode(a);
XmlAttribute b = doc.CreateAttribute("编号");
b.InnerText = Convert.ToString(_bookmodel.BookID);
xelKey.SetAttributeNode(b);
XmlElement c = doc.CreateElement("书名");
c.InnerText = _bookmodel.BookName;
xelKey.AppendChild(c);
XmlElement d = doc.CreateElement("作者");
d.InnerText = _bookmodel.BookAuthor;
xelKey.AppendChild(d);
XmlElement e = doc.CreateElement("价格");
e.InnerText = _bookmodel.BookPrice;
xelKey.AppendChild(e);
root.AppendChild(xelKey);
doc.Save("Book.xml");
} //增加
public void DeleteBookInfo(string a)
{
XElement xe = XElement.Load("Book.xml");
IEnumerable<XElement> elements = from ele in xe.Elements("book")
where ele.Attribute("编号").Value == a
select ele;
if (elements.Count() > 0)
{
elements.First().Remove();
}
xe.Save("Book.xml");
} //删除
public void UpdateBookInfo(BookModel a)
{
XElement xe = XElement.Load("Book.xml");
IEnumerable<XElement> element = from ele in xe.Elements("book")
where ele.Attribute("编号").Value == Convert.ToString(a.BookID)
select ele;
if (element.Count() > 0)
{
XElement first = element.First();
//设置新的属性
first.SetAttributeValue("课程类型", a.BookType);
first.SetAttributeValue("编号", a.BookID);
//替换新的节点
first.ReplaceNodes(
new XElement("书名", a.BookName),
new XElement("作者", a.BookAuthor),
new XElement("价格", a.BookPrice)
);
}
xe.Save("Book.xml");
} //更改
public List<BookModel> QueryBookInfo(int intFalg,string c)
{
List<BookModel> bookList = new List<BookModel>();
XElement xml = XElement.Load(strPath);
var bookVar = xml.Descendants("book"); //默认查询所有图书
switch (intFalg) {
case 1: //"根据编号查询"
bookVar = xml.Descendants("book").Where(a => a.Attribute("编号").Value ==c); //c由UI端写入数据传到这儿
break;
case 2://"根据书名查询"
bookVar = xml.Descendants("book").Where(a => a.Element("书名").Value == c);
break;
case 3://"根据作者查询"
bookVar = xml.Descendants("book").Where(a => a.Element("作者").Value == c);
break;
}
bookList = (from book in bookVar
select new BookModel
{
BookID = int.Parse(book.Attribute("编号").Value),
BookType = book.Attribute("课程类型").Value,
BookName = book.Element("书名").Value,
BookAuthor = book.Element("作者").Value,
BookPrice = book.Element("价格").Value,
}).ToList();
return bookList;
} //查询
}
}
BLL(业务逻辑层)代码:
using OperationXML.DAL;
using OperationXML.Model;
using System.Collections.Generic;
using System.Data;
namespace OperationXML.BLL
{
public class BookBll
{
BookModel _person= new BookModel();
BookDal _a = new BookDal();
public DataSet PersonLoad()
{
DataSet ds = _a.LoadBookInfo();
return ds;
} //加载
public void Creat(BookModel n)
{
_a.CreatBookInfo(n);
} //创建
public void Add(BookModel m)
{
_a.AddBookInfo(m);
} //添加
public void Delete(string _bookmodel)
{
_a.DeleteBookInfo(_bookmodel);
} //删除
public void Update(BookModel d)
{
_a.UpdateBookInfo(d);
} //更改
public List<BookModel> Query( int intFalg,string c)
{
List<BookModel> list = new List<BookModel>();
list = _a.QueryBookInfo(intFalg,c);
return list;
} //查询
}
}
Add.cs类
using OperationXML.BLL;
using OperationXML.Model;
using System;
using System.Windows.Forms;
namespace OperationXML.UI
{
public partial class Add : Form
{
BookBll _bbb = new BookBll();
BookModel _book = new BookModel();
public Add()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
_book.BookType = this.comboBox1.Text;
_book.BookPrice = this.txtPrice.Text;
_book.BookID = Convert.ToInt32(txtISBN.Text);
_book.BookName = this.txtName.Text;
_book.BookAuthor = this.txtAuthor.Text;
_bbb.Add(_book);
Close();
}
private void button2_Click(object sender, EventArgs e)
{
Close();
}
}
}
using OperationXML.BLL;
using OperationXML.Model;
using System;
using System.Windows.Forms;
namespace OperationXML.UI
{
public partial class CreatXML : Form
{
public CreatXML()
{
InitializeComponent();
}
BookModel _book = new BookModel();
BookBll _b = new BookBll();
private void button1_Click(object sender, EventArgs e)
{
_book.BookName = txtTitle.Text;
_book.BookAuthor = txtauthor.Text;
_book.BookPrice = txtPrice.Text;
_book.BookID = Convert.ToInt32(txtID.Text);
_book.BookType = comboBox1.Text;
_b.Creat(_book);
Close();
}
private void button2_Click(object sender, EventArgs e)
{
Close();
}
}
}
using OperationXML.BLL;
using OperationXML.Model;
using System;
using System.Collections.Generic;
using System.Data;
using System.Windows.Forms;
namespace OperationXML.UI
{
public partial class Form1 : Form
{
BookModel _bookmodel = new BookModel();
BookBll _b = new BookBll();
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
CreatXML cx = new CreatXML();
cx.ShowDialog();
}
private void button2_Click(object sender, EventArgs e)
{
DataSet ds = _b.PersonLoad();
dataGridView1.DataSource = ds.Tables[0];
for (int i = 0; i < dataGridView1.RowCount; i )
{
dataGridView1.Rows[i].Height = 50;
}
for (int i = 0; i < dataGridView1.ColumnCount; i )
{
dataGridView1.Columns[i].Width = 200;
}
}
private void button3_Click(object sender, EventArgs e)
{
Add _add = new Add();
_add.ShowDialog();
}
private void button4_Click(object sender, EventArgs e)
{
DataGridViewRow _Row = dataGridView1.CurrentRow;
DataGridViewSelectedRowCollection drArray = dataGridView1.SelectedRows;
if (_Row == null)
{
MessageBox.Show("没有数据行,操作无效!");
return; //要问的问题
}
List<string> list = new List<string>();
foreach (DataGridViewRow singleRow in drArray)
{
list.Add(singleRow.Cells["编号"].Value.ToString());
}
if (MessageBox.Show("确定删除此行数据?", "Confirmation", MessageBoxButtons.OKCancel) == DialogResult.OK)
{
foreach (string s in list)
{
_b.Delete(s);
}
MessageBox.Show("删除数据成功!");
}
}
private void button5_Click(object sender, EventArgs e)
{
Query qe = new Query();
qe.ShowDialog();
}
private void dataGridView1_MouseDoubleClick(object sender, MouseEventArgs e)
{
DataGridViewRow _Row = dataGridView1.CurrentRow;
if (_Row == null)
{
MessageBox.Show("没有数据行,当前操作无效!");
return;
}
Modify md = new Modify();
md.CurrentRow = _Row;
md.ShowDialog();
}
}
}
using OperationXML.BLL;
using OperationXML.Model;
using System;
using System.Windows.Forms;
namespace OperationXML.UI
{
public partial class Modify : Form
{
BookBll _b = new BookBll();
BookModel _book = new BookModel();
private DataGridViewRow _CurrentRow;
public DataGridViewRow CurrentRow
{
get { return _CurrentRow; }
set { _CurrentRow = value; }
}
public Modify()
{
InitializeComponent();
}
private void Modify_Load(object sender, EventArgs e) //加载到页面
{
txtAuthor.Text = _CurrentRow.Cells["作者"].Value.ToString();
txtID.Text = _CurrentRow.Cells["编号"].Value.ToString();
txtName.Text = _CurrentRow.Cells["书名"].Value.ToString();
txtPrice.Text = _CurrentRow.Cells["价格"].Value.ToString();
comboBox1.Text = _CurrentRow.Cells["课程类型"].Value.ToString();
}
private void button1_Click(object sender, EventArgs e)
{
_book.BookAuthor = txtAuthor.Text;
_book.BookID = Convert.ToInt32(txtID.Text);
_book.BookName = txtName.Text;
_book.BookPrice = txtPrice.Text;
_book.BookType = comboBox1.Text;
_b.Update(_book);
Close();
}
private void button2_Click(object sender, EventArgs e)
{
Close();
}
}
}
using OperationXML.BLL;
using OperationXML.Model;
using System;
using System.Collections.Generic;
using System.Windows.Forms;
namespace OperationXML.UI
{
public partial class Query : Form
{
BookBll _b = new BookBll(); //实例化(调用)B层
BookModel _bookmodel = new BookModel(); //实例化(调用)Model层
List<BookModel> list = null;
public Query()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
string _str = comboBox1.Text;
if (_str == "")
{
MessageBox.Show("请选择查询条件!");
return;
}
if (textBox1.Text == "")
{
MessageBox.Show("请输入查询条件!");
return;
}
switch (_str)
{
case "编号":
list = _b.Query(1, textBox1.Text);
break;
case "书名":
list = _b.Query(2, textBox1.Text);
break;
case "作者":
list = _b.Query(3, textBox1.Text);
break;
default:
break;
}
dataGridView1.DataSource = list;
for (int i = 0; i < dataGridView1.RowCount; i )
{
dataGridView1.Rows[i].Height = 50;
} //设置行高
for (int i = 0; i < dataGridView1.ColumnCount; i )
{
dataGridView1.Columns[i].Width = 160;
} //设置列宽
}
private void button2_Click(object sender, EventArgs e)
{
Close();
}
}
}
以上就是本案例所有的代码了,用的是3层结构做的,这是一个很简单的开发案例,不足之处,希望另有学者提出宝贵意见,谢谢大家!
小贴士
感谢您为本站写下的评论,您的评论对其它用户来说具有重要的参考价值,所以请认真填写。
- 类似“顶”、“沙发”之类没有营养的文字,对勤劳贡献的楼主来说是令人沮丧的反馈信息。
- 相信您也不想看到一排文字/表情墙,所以请不要反馈意义不大的重复字符,也请尽量不要纯表情的回复。
- 提问之前请再仔细看一遍楼主的说明,或许是您遗漏了。
- 请勿到处挖坑绊人、招贴广告。既占空间让人厌烦,又没人会搭理,于人于己都无利。
关于好例子网
本站旨在为广大IT学习爱好者提供一个非营利性互相学习交流分享平台。本站所有资源都可以被免费获取学习研究。本站资源来自网友分享,对搜索内容的合法性不具有预见性、识别性、控制性,仅供学习研究,请务必在下载后24小时内给予删除,不得用于其他任何用途,否则后果自负。基于互联网的特殊性,平台无法对用户传输的作品、信息、内容的权属或合法性、安全性、合规性、真实性、科学性、完整权、有效性等进行实质审查;无论平台是否已进行审查,用户均应自行承担因其传输的作品、信息、内容而可能或已经产生的侵权或权属纠纷等法律责任。本站所有资源不代表本站的观点或立场,基于网友分享,根据中国法律《信息网络传播权保护条例》第二十二与二十三条之规定,若资源存在侵权或相关问题请联系本站客服人员,点此联系我们。关于更多版权及免责申明参见 版权及免责申明
网友评论
我要评论