实例介绍
此案例用的是
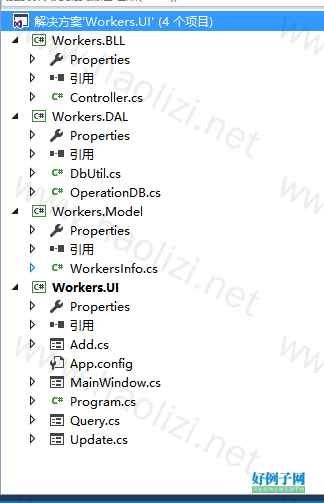

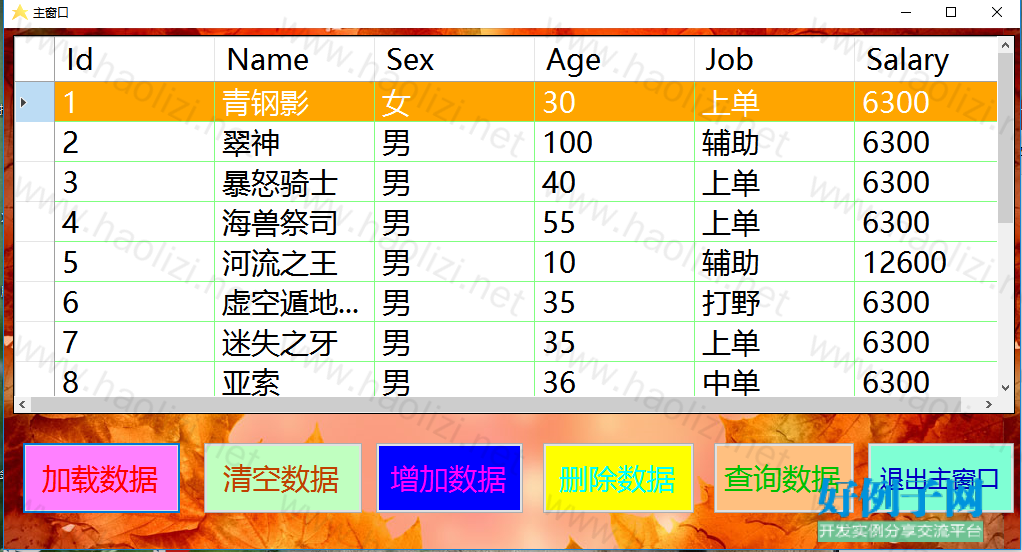

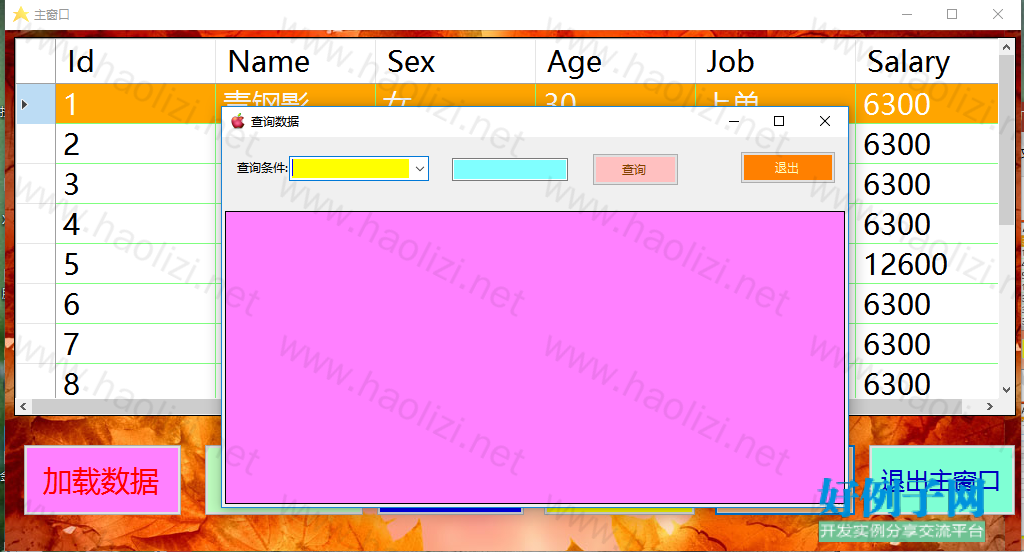
【核心代码】
Model层代码:
namespace Workers.Model
{
public class WorkersInfo
{
private int _Id;
public int Id
{
get { return _Id; }
set { _Id = value; }
}
private string _Name;
public string Name
{
get { return _Name; }
set { _Name = value; }
}
private string _Sex;
public string Sex
{
get { return _Sex; }
set { _Sex = value; }
}
private string _Age;
public string Age
{
get { return _Age; }
set { _Age = value; }
}
private string _Job;
public string Job
{
get { return _Job; }
set { _Job = value; }
}
private int _Salary;
public int Salary
{
get { return _Salary; }
set { _Salary = value; }
}
}
}
DAL层代码:
using System.Data.SqlClient;
namespace Workers.DAL
{
public class DbUtil
{
SqlConnection conn;
public static string ConnStr = "server=.;database=Work1;uid=sa;pwd=";
public SqlConnection GetConn()
{
conn = new SqlConnection(ConnStr);
conn.Open();
return conn;
}
}
}
using System;
using System.Data;
using System.Data.SqlClient;
using System.Windows.Forms;
using Workers.Model;
namespace Workers.DAL
{
public class OperationDB
{
DbUtil db = new DbUtil();
SqlConnection conn;
SqlCommand cmd;
SqlDataAdapter sda;
#region 加载
public DataSet Load()
{
sda = new SqlDataAdapter("select * from tb_workers2", db.GetConn());
DataSet ds = new DataSet();
sda.Fill(ds);
return ds;
}
#endregion
#region 添加
public void Add(WorkersInfo Info)
{
try
{
string str_Add = "insert into tb_workers2 values('"
Info.Id "','" Info.Name "','" Info.Sex "','"
Info.Age "','" Info.Job "','" Info.Salary "')";
conn = db.GetConn();
cmd = new SqlCommand(str_Add, conn);
cmd.ExecuteNonQuery();
conn.Dispose(); //conn.close();
}
catch (Exception ee)
{
MessageBox.Show(ee.ToString());
}
}
#endregion
#region 删除
public void Delete(string a)
{
try
{
string str_Delete = "delete from tb_workers2 where Id=" a;
conn = db.GetConn();
cmd = new SqlCommand(str_Delete, conn);
cmd.ExecuteNonQuery();
conn.Dispose();
}
catch (Exception ee)
{
MessageBox.Show(ee.ToString());
}
}
#endregion
#region 修改
public void Update(WorkersInfo Info)
{
try
{
string str_update = "update tb_workers2 set ";
str_update = "Name='" Info.Name "',Sex='" Info.Sex "',";
str_update = "Age='" Info.Age "',Job='" Info.Job "',Salary='" Info.Salary "'";
str_update = "where Id=" Info.Id;
conn = db.GetConn();
cmd = new SqlCommand(str_update, conn);
cmd.ExecuteNonQuery();
conn.Dispose();
}
catch (Exception ee)
{
MessageBox.Show(ee.ToString());
}
}
#endregion
#region 查询
public DataSet Find(string strObject, int intFalg)
{
string strSecar = null;
try
{
switch (intFalg)//判断条件
{
case 1://"Id"
strSecar = "select * from tb_workers2 where Id like'" strObject "%'";
//strSecar = string.Format("select * from tb_workers where 姓名 like'{0}%'",this.textBox1.Text.Trim());
break;
case 2://"Sex"
strSecar = "select * from tb_workers2 where Sex like'" strObject "%'";
break;
case 3://"Age":
strSecar = "select * from tb_workers2 where Age like'" strObject "%'";
break;
case 4://"Job"
strSecar = "select * from tb_workers2 where Job like'" strObject "%'";
break;
}
DataSet ds = new DataSet();
conn = db.GetConn();
cmd = new SqlCommand(strSecar, conn);
sda = new SqlDataAdapter();
sda.SelectCommand = cmd;
sda.Fill(ds);
return ds;
}
catch (Exception ee)
{
MessageBox.Show(ee.Message);
return null;
}
}
#endregion
}
}
using System.Data;
using Workers.DAL;
using Workers.Model;
namespace Workers.BLL
{
public class Controller
{
OperationDB _OperationDB = new OperationDB();
public DataSet WorkersLoad()
{
DataSet ds = _OperationDB.Load();
return ds;
} //加载
public void WorkersAdd(WorkersInfo add)
{
_OperationDB.Add(add);
}//添加
public void WorkersDelete(string b)
{
_OperationDB.Delete(b);
}//删除
public void WorkersUpdate(WorkersInfo update)
{
_OperationDB.Update(update);
}//更改
public DataSet WorkersFind(string strObject, int intFalg)
{
DataSet ds = _OperationDB.Find(strObject, intFalg);
return ds;
}//查询
}
}
Add.cs代码:
using System;
using System.Windows.Forms;
using Workers.BLL;
using Workers.Model;
namespace Workers.UI
{
public partial class Add : Form
{
public Add()
{
InitializeComponent();
}
Controller _Controller = new Controller();
private void button1_Click(object sender, EventArgs e)
{
WorkersInfo w = new WorkersInfo();
w.Id = int.Parse(textBox1.Text);
w.Name = textBox2.Text;
w.Sex = textBox3.Text;
w.Age = textBox4.Text;
w.Job = textBox5.Text;
w.Salary = int.Parse(textBox6.Text);
if (MessageBox.Show("确定添加到数据库?", "Confirmation", MessageBoxButtons.OKCancel) == DialogResult.OK)
{
_Controller.WorkersAdd(w);
Close();
}
}
private void button2_Click(object sender, EventArgs e)
{
Close();
}
private void button3_Click(object sender, EventArgs e)
{
textBox1.Text = textBox2.Text = textBox3.Text = textBox4.Text = textBox5.Text = textBox6.Text = null;
}
}
}
using System.Collections.Generic;
using System.Data;
using System.Drawing;
using System.Windows.Forms;
using Workers.BLL;
namespace Workers.UI
{
public partial class MainWindow : Form
{
public MainWindow()
{
InitializeComponent();
}
Controller _Controller = new Controller();
private void button1_Click(object sender, EventArgs e)
{
DataSet ds = _Controller.WorkersLoad();
dataGridView1.DataSource = ds.Tables[0];
for (int j = 0; j < dataGridView1.RowCount; j )
{
dataGridView1.Rows[j].Height = 40;
} //设置 dataGridView控件行的高度
for (int i = 0; i < dataGridView1.ColumnCount; i )
{
dataGridView1.Columns[i].Width = 160;
}//设置dataGridView控件行的宽度
//选中行的背景色
dataGridView1.DefaultCellStyle.SelectionBackColor = Color.Orange;
}
private void button2_Click(object sender, EventArgs e)
{
Add add = new Add();
add.ShowDialog();
}
private void button3_Click(object a, EventArgs e)
{
DataGridViewRow _Row = dataGridView1.CurrentRow;
DataGridViewSelectedRowCollection selectedrows = dataGridView1.SelectedRows;
if (_Row == null)
{
MessageBox.Show("没有数据行,操作无效!");
return; //要问的问题
}
List<string> list = new List<string>();
foreach (DataGridViewRow singleRow in selectedrows)
{
list.Add(singleRow.Cells["Id"].Value.ToString());
}
if (MessageBox.Show("确定删除此行数据?", "Confirmation", MessageBoxButtons.OKCancel) == DialogResult.OK)
{
foreach (string s in list)
{
_Controller.WorkersDelete(s);
}
MessageBox.Show("删除数据成功!");
}
}
private void button5_Click(object sender, EventArgs e)
{
Query qe = new Query();
qe.ShowDialog();
}
private void button4_Click(object sender, EventArgs e)
{
Close();
}
private void dataGridView1_MouseDoubleClick(object sender, MouseEventArgs e)
{
DataGridViewRow _Row = dataGridView1.CurrentRow;
if (_Row == null)
{
MessageBox.Show("没有数据行,当前操作无效!");
return;
}
Update up = new Update();
up.CurrentRow = _Row; // _Row==>up.CurrentRow(属性)
up.ShowDialog();
}
private void button6_Click(object sender, EventArgs e)
{
dataGridView1.DataSource = null;
}
}
}
using System;
using System.Data;
using System.Drawing;
using System.Windows.Forms;
using Workers.BLL;
namespace Workers.UI
{
public partial class Query : Form
{
Controller _Controller = new Controller();
DataSet ds = null;
public Query()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
string P_Str_selectcondition = comboBox1.Text;
if (P_Str_selectcondition == "")
{
MessageBox.Show("请选择查询条件!");
return;
}
if (textBox1.Text == "")
{
MessageBox.Show("请输入查询条件!");
return;
}
switch (P_Str_selectcondition)
{
case "Id":
ds = _Controller.WorkersFind(textBox1.Text, 1);
break;
case "Sex":
ds = _Controller.WorkersFind(textBox1.Text, 2);
break;
case "Age":
ds = _Controller.WorkersFind(textBox1.Text, 3);
break;
case "Job":
ds = _Controller.WorkersFind(textBox1.Text, 4);
break;
default:
break;
}
dataGridView1.DataSource = ds.Tables[0];
for (int j = 0; j < dataGridView1.RowCount; j )
{
dataGridView1.Rows[j].Height = 40;
}
for (int i = 0; i < dataGridView1.ColumnCount; i )
{
dataGridView1.Columns[i].Width = 100;
}
dataGridView1.DefaultCellStyle.SelectionBackColor = Color.Orange;
}
private void button2_Click(object sender, EventArgs e)
{
Close();
}
}
}
using System;
using System.Windows.Forms;
using Workers.BLL;
using Workers.Model;
namespace Workers.UI
{
public partial class Update : Form
{
public Update()
{
InitializeComponent();
}
Controller _Controller = new Controller();
private DataGridViewRow _CurrentRow;
public DataGridViewRow CurrentRow
{
get { return _CurrentRow; }
set { _CurrentRow = value; }
}
private void button1_Click(object sender, EventArgs e)
{
WorkersInfo d = new WorkersInfo();
d.Id = int.Parse(text_ID.Text);
d.Name = text_Name.Text;
d.Sex = text_Sex.Text;
d.Age = text_Age.Text;
d.Job = text_Job.Text;
d.Salary = int.Parse(text_Salary.Text);
if (MessageBox.Show("确定修改到数据库?", "Confirmation", MessageBoxButtons.OKCancel) == DialogResult.OK)
{
_Controller.WorkersUpdate(d);
Close();
}
}
private void button2_Click(object sender, EventArgs e)
{
Close();
}
private void Update_Load(object sender, EventArgs e)
{
text_ID.Text = _CurrentRow.Cells["Id"].Value.ToString();
text_Name.Text = _CurrentRow.Cells["Name"].Value.ToString();
text_Sex.Text = _CurrentRow.Cells["Sex"].Value.ToString();
text_Age.Text = _CurrentRow.Cells["Age"].Value.ToString();
text_Job.Text = _CurrentRow.Cells["Job"].Value.ToString();
text_Salary.Text = _CurrentRow.Cells["Salary"].Value.ToString();
} //更新窗口加载时,就给了text赋了值
}
}
相关软件
小贴士
感谢您为本站写下的评论,您的评论对其它用户来说具有重要的参考价值,所以请认真填写。
- 类似“顶”、“沙发”之类没有营养的文字,对勤劳贡献的楼主来说是令人沮丧的反馈信息。
- 相信您也不想看到一排文字/表情墙,所以请不要反馈意义不大的重复字符,也请尽量不要纯表情的回复。
- 提问之前请再仔细看一遍楼主的说明,或许是您遗漏了。
- 请勿到处挖坑绊人、招贴广告。既占空间让人厌烦,又没人会搭理,于人于己都无利。
关于好例子网
本站旨在为广大IT学习爱好者提供一个非营利性互相学习交流分享平台。本站所有资源都可以被免费获取学习研究。本站资源来自网友分享,对搜索内容的合法性不具有预见性、识别性、控制性,仅供学习研究,请务必在下载后24小时内给予删除,不得用于其他任何用途,否则后果自负。基于互联网的特殊性,平台无法对用户传输的作品、信息、内容的权属或合法性、安全性、合规性、真实性、科学性、完整权、有效性等进行实质审查;无论平台是否已进行审查,用户均应自行承担因其传输的作品、信息、内容而可能或已经产生的侵权或权属纠纷等法律责任。本站所有资源不代表本站的观点或立场,基于网友分享,根据中国法律《信息网络传播权保护条例》第二十二与二十三条之规定,若资源存在侵权或相关问题请联系本站客服人员,点此联系我们。关于更多版权及免责申明参见 版权及免责申明
网友评论
我要评论